Time is a critical element in software development, and handling it effectively is essential for building robust and reliable applications. In the world of Java programming, one library stands out for simplifying date and time operations – Joda Time. In this article, we will delve deep into Joda Time, exploring its features, benefits, and how to use it to conquer the complexities of date and time in your Java projects.
Joda Time is an open-source Java library designed to replace the inadequate date and time classes available in the Java standard library (java.util.Date and java.util.Calendar). It offers a more intuitive and comprehensive way to work with dates, times, durations, and intervals. Joda Time was created by Stephen Colebourne and has become the go-to solution for handling date and time operations in Java applications.
Key Features of Joda Time
Joda Time is a powerful date and time library for Java that offers a wide range of features and capabilities. In this comprehensive overview, we’ll delve into its key features, providing detailed explanations and examples. These features include immutability, comprehensive date and time types, a fluent API, extensibility, time zone support, and parsing and formatting capabilities.
1. Immutable and Thread-Safe
Joda Time objects are immutable and thread-safe. This fundamental feature ensures data consistency and prevents race conditions in multithreaded applications. Once you create an instance of a Joda Time object, such as LocalDate, LocalTime, or LocalDateTime, you cannot alter its state. This immutability guarantees that the object’s value remains unchanged throughout its lifetime, making it ideal for concurrent programming.
2. Comprehensive Date and Time Types
Joda Time offers a rich set of classes to represent various aspects of date and time:
Type | Description | Example |
---|---|---|
LocalDate | Represents a date without a time zone. | “2023-09-24” |
LocalTime | Represents a time without a date. | “14:30:00” |
LocalDateTime | Combines a date and a time. | “2023-09-24T14:30:00” |
DateTime | Represents a date and time with a time zone. | “2023-09-24T14:30:00Z” |
Period | Represents a time duration in years, months, days, hours, minutes, and seconds. | 2 years, 3 months, 7 days |
Interval | Represents a time span between two points in time. | 2023-09-24T14:30:00 to 2023-09-30T12:00:00 |
These classes cover a wide range of date and time scenarios, allowing you to work with precision in your applications.
3. Fluent API
Joda Time provides a fluent and intuitive API for manipulating date and time objects. The fluent API allows you to chain methods together to create concise and readable code. For instance, you can easily perform operations like adding days, weeks, or months to a date:
LocalDate now = LocalDate.now(); LocalDate nextWeek = now.plusWeeks(1); |
This example demonstrates how you can fluently add one week to the current date, resulting in nextWeek being set to the date exactly one week from now.
4. Extensible and Customizable
Joda Time is designed to be extensible and customizable to meet your specific requirements. You can create custom date and time fields or chronologies, making it suitable for handling non-Gregorian calendars or unconventional date formats. This extensibility empowers developers to adapt Joda Time to various cultural and domain-specific needs.
5. Time Zone Support
Handling time zones correctly is often challenging, but Joda Time simplifies time zone management. It offers robust support for time zones, including:
- Conversions between different time zones;
- Handling daylight saving time adjustments;
- Ensuring accurate and reliable time zone calculations.
With Joda Time, you can confidently work with date and time data in different parts of the world without worrying about complex time zone issues.
6. Parsing and Formatting
Joda Time provides powerful parsing and formatting capabilities. It enables you to convert between text representations of date and time and Joda Time objects effortlessly. You can define custom date and time patterns or use predefined formats, making it easy to process date and time data from various sources.
Getting Started with Joda Time
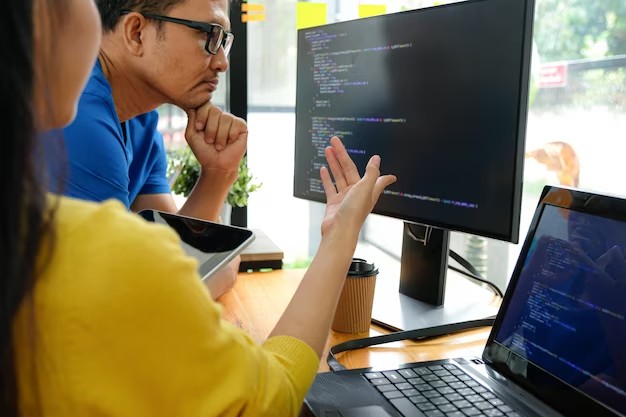
Now that we’ve covered the key features of Joda Time let’s explore how to get started with this powerful library:
1. Adding Joda Time to Your Project
To use Joda Time in your Java project, you need to add the Joda Time library to your project’s classpath. You can do this manually by downloading the JAR file from the official website or by using build tools like Maven or Gradle.
For Maven users, add the following dependency to your pom.xml:
<dependency> <groupId>joda-time</groupId> <artifactId>joda-time</artifactId> <version>2.10.13</version> <!– Use the latest version –> </dependency> |
For Gradle users, add the following to your build.gradle:
dependencies { implementation ‘joda-time:joda-time:2.10.13’ // Use the latest version } |
2. Creating Joda Time Objects
Once you’ve added Joda Time to your project, you can start using its classes to work with date and time. Here’s how you can create some common Joda Time objects:
Creating a LocalDate:
LocalDate date = LocalDate.of(2023, 9, 24); |
Creating a LocalTime:
LocalTime time = LocalTime.of(14, 30); |
Creating a LocalDateTime:
LocalDateTime dateTime = LocalDateTime.of(2023, 9, 24, 14, 30); |
Creating a DateTime with a Time Zone:
DateTime dateTimeWithTimeZone = new DateTime(2023, 9, 24, 14, 30, DateTimeZone.UTC); |
3. Performing Operations
Joda Time simplifies date and time operations. Here are some common operations you might need:
Adding/Subtracting Duration:
LocalDate today = LocalDate.now(); LocalDate tomorrow = today.plusDays(1); LocalDate yesterday = today.minusDays(1); |
Comparing Dates:
LocalDate date1 = LocalDate.of(2023, 9, 24); LocalDate date2 = LocalDate.of(2023, 9, 25); boolean isAfter = date1.isAfter(date2); // false boolean isBefore = date1.isBefore(date2); // true boolean isEqual = date1.isEqual(date2); // false |
Formatting Dates:
DateTimeFormatter formatter = DateTimeFormat.forPattern(“yyyy-MM-dd”); String formattedDate = formatter.print(date); // “2023-09-24” |
Parsing Dates:
String dateStr = “2023-09-24”; LocalDate parsedDate = LocalDate.parse(dateStr, formatter); |
4. Time Zone Handling
Joda Time makes working with time zones straightforward. You can easily convert between time zones and handle daylight saving time transitions:
Converting Between Time Zones:
DateTime dateTime = new DateTime(2023, 9, 24, 14, 30, DateTimeZone.UTC); DateTime dateTimeInNewYork = dateTime.withZone(DateTimeZone.forID(“America/New_York”)); |
Handling Daylight Saving Time:
DateTime dateTime = new DateTime(2023, 3, 12, 2, 0, DateTimeZone.forID(“America/New_York”)); DateTime adjustedDateTime = dateTime.plusHours(1); |
Conclusion
Joda Time is a powerful and flexible Java library that simplifies date and time handling in your applications. Its comprehensive set of classes, fluent API, and time zone support make it a valuable tool for developers. Whether you’re dealing with simple date calculations or complex internationalization requirements, Joda Time can help you conquer the challenges of managing time effectively in your Java projects. So why wait? Start using Joda Time today and unlock the full potential of date and time manipulation in your Java applications.
FAQs
Joda Time was still actively maintained, but it was considered a stable library with few major updates. However, the landscape of Java libraries can change over time.
Java 8 introduced a new Date and Time API (java.time) that addressed many of the shortcomings of the old java.util.Date and java.util.Calendar classes. If you are using Java 8 or later, it is recommended to use the java.time API, as it is part of the Java standard library and benefits from ongoing support and improvements. However, if you are working with older versions of Java, Joda Time remains an excellent choice for handling date and time operations.
Yes, Joda Time is designed to be extensible, and you can create custom chronologies to work with non-Gregorian calendars. It provides a flexible framework for handling different calendar systems, making it a versatile choice for internationalization and localization.
Joda Time was initially developed before the introduction of Java modules in Java 9. While it should work in Java 9 and later versions, there may be some considerations regarding module compatibility. Ensure that you consult the official documentation and update your project configuration as needed to make Joda Time work seamlessly with Java modules.
Joda Time, like most date and time libraries, does not handle leap seconds. Leap seconds are adjustments made to Coordinated Universal Time (UTC) and are typically handled at the system level rather than within application code. When precise handling of leap seconds is required, it’s advisable to work with specialized time libraries designed for such purposes.