Java, a versatile and widely used programming language, offers developers various tools and functions to manipulate data efficiently. Among these, strings are essential for handling text and character data. However, it’s crucial to understand the memory usage of Java strings, as it directly impacts the performance and resource consumption of your Java applications. In this article, we will delve deep into the concept of Java string byte size, exploring its significance, how it works, and best practices for managing it effectively.
The Significance of Java String Byte Size
Java strings are objects that represent sequences of characters. These characters can be letters, numbers, symbols, or even whitespace. Strings are used extensively in Java applications for tasks such as storing user input, processing text files, and communicating with external systems.
One key aspect of Java strings is their memory consumption, often measured in bytes. The memory usage of a string is vital because it determines how much memory your program will allocate to store string data. Inefficient use of memory can lead to performance issues and even application crashes if memory resources are exhausted.
Understanding and optimizing the byte size of Java strings is crucial for the following reasons:
- Memory Efficiency: By managing the byte size of strings effectively, you can reduce the memory footprint of your Java applications, making them more efficient and responsive;
- Performance: Smaller strings consume less memory and are processed more quickly, leading to improved application performance;
- Resource Management: Properly managing string memory usage helps prevent memory leaks and ensures your application runs smoothly without consuming excessive resources.
How Java Stores Strings in Memory
To comprehend the Java string byte size, it’s crucial to delve into how Java stores strings in memory. In the realm of Java programming, strings are typically realized as objects of the java.lang.String class. These objects are structured into two principal components:
- Character Array: The core of a Java string is a character array (char[]), residing within the String object. Each character in this array generally occupies 2 bytes of memory. Java employs the UTF-16 encoding, which extends character representation to accommodate a wide range of international characters and symbols. UTF-16 encoding assigns 2 bytes to each character in the character array, ensuring compatibility for multilingual applications.
To illustrate, consider the string “Java,” which consists of four characters (‘J’, ‘a’, ‘v’, ‘a’). When stored in memory, this string occupies 8 bytes (4 characters × 2 bytes per character); - Additional Metadata: Apart from the character array, the String object contains essential metadata. This metadata encompasses information like the length of the string and various methods for performing operations on the string. These are crucial for efficiently manipulating and accessing the string’s content.
Now, let’s dive deeper into calculating the byte size of a Java string:
Byte Size Calculation
To determine the byte size of a Java string, you can employ the following formula:
Byte Size = 2 * (number of characters) + 38 |
In this formula, the 2 * (number of characters) segment accounts for the memory required to store the characters in the character array. Each character consumes 2 bytes, as previously explained. Additionally, there is an overhead of +38 bytes, which covers the metadata and object-related memory usage.
Example Calculation
Let’s illustrate this calculation with an example. Suppose you have a Java string: “OpenAI.” This string consists of 6 characters (‘O’, ‘p’, ‘e’, ‘n’, ‘A’, ‘I’). Applying the formula:
Byte Size = 2 * 6 + 38 Byte Size = 12 + 38 Byte Size = 50 bytes |
Hence, the Java string “OpenAI” consumes 50 bytes of memory.
Factors Affecting Java String Byte Size
The byte size of a Java string depends on several factors, and understanding these factors is crucial for efficient memory management in your Java applications. Here are the main factors that influence Java string byte size:
1. Number of Characters
The most significant factor affecting the byte size of a Java string is the number of characters it contains. As mentioned earlier, each character typically occupies 2 bytes in memory due to UTF-16 encoding. Therefore, longer strings consume more memory than shorter ones.
2. Encoding
While UTF-16 is the default encoding for Java strings, you can choose other encodings, such as UTF-8, when working with byte arrays or streams. Keep in mind that using a different encoding may affect the byte size of your strings, as different encodings use varying numbers of bytes to represent characters.
3. Special Characters
Certain characters, such as non-ASCII characters, symbols, or emojis, may require more than 2 bytes in memory. This is because they may be represented as surrogate pairs in UTF-16 encoding, which increases their memory footprint.
4. String Pool
Java maintains a string pool (also known as the string intern pool) for storing string literals. Strings stored in the pool can be reused, which can save memory. However, this can also lead to unexpected memory usage if you’re not careful, as strings in the pool are kept in memory until the application exits or they are explicitly removed.
5. Concatenation
When you concatenate strings in Java using the + operator or the concat() method, a new string object is created, which may result in increased memory usage. To mitigate this, you can use StringBuilder or StringBuffer for efficient string concatenation.
6. Substrings
Creating substrings from a larger string does not create a new character array. Instead, it creates a new String object that references the original character array. This can lead to increased memory usage if you retain a reference to the original string.
Best Practices for Managing Java String Byte Size
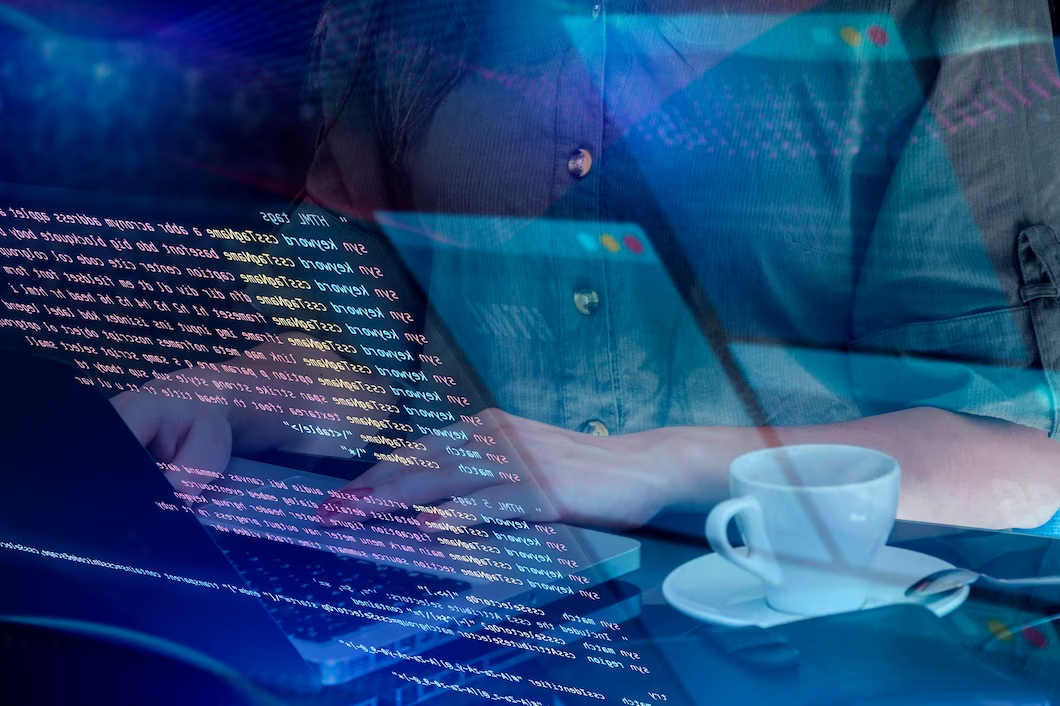
To optimize the memory usage of Java strings and ensure your applications perform efficiently, consider the following best practices:
1. Use StringBuilder for String Concatenation
When you need to concatenate multiple strings, use the StringBuilder class instead of the + operator or concat() method. StringBuilder is more memory-efficient as it minimizes the creation of intermediate string objects.
StringBuilder builder = new StringBuilder(); builder.append(“Hello”); builder.append(” “); builder.append(“World”); String result = builder.toString(); |
2. Be Mindful of String Pool Usage
While the string pool can help save memory for repeated string literals, be cautious when adding large or dynamically generated strings to the pool. You can use the intern() method to add a string to the pool explicitly.
String dynamicString = “Dynamic String”; String internedString = dynamicString.intern(); |
3. Limit the Use of Non-ASCII Characters
If your application deals with non-ASCII characters extensively, be aware that they may consume more memory due to UTF-16 encoding. Consider using encodings like UTF-8 if possible, as they may result in smaller byte sizes for non-ASCII characters.
4. Avoid Unnecessary Substring References
Be mindful when working with substrings. If you create a substring but no longer need the original string, release the reference to it. Otherwise, you may inadvertently hold on to more memory than necessary.
5. Monitor Memory Usage
Use Java profiling and monitoring tools to keep an eye on your application’s memory usage. This will help you identify memory leaks and areas where memory optimization is needed.
Conclusion
Understanding and managing Java string byte size is crucial for developing efficient and resource-friendly Java applications. By optimizing the memory usage of your strings, you can improve the performance and reliability of your software. Remember to consider factors like the number of characters, encoding, and string pool usage when working with strings in Java. By following best practices and being mindful of memory consumption, you can create Java applications that are both powerful and memory-efficient.
FAQs
The default encoding for Java strings is UTF-16, which typically uses 2 bytes to represent each character.
You can convert a string to bytes in Java using the getBytes() method, which allows you to specify the encoding. For example:
String myString = “Hello, World!”;
byte[] bytes = myString.getBytes(StandardCharsets.UTF_8);
You can convert a string from one encoding to another by first converting it to bytes using one encoding and then decoding those bytes using another encoding. However, it’s important to handle encoding conversions carefully to avoid data loss.
Both StringBuilder and StringBuffer are used for efficient string manipulation, but StringBuilder is not thread-safe, while StringBuffer is. Use StringBuilder in single-threaded scenarios for better performance and StringBuffer in multi-threaded environments.
You can calculate the byte size of a string in Java using the formula: 2 * (number of characters) + 38. This formula accounts for the memory required to store the characters and the additional metadata.