In the realm of Java programming, the long data type is well-known for its ability to store large integers. But what happens when you need to map one long to another? Enter “Java Long Long.” In this article, we will delve into the world of Java Long Long, explore its use cases, and learn how to efficiently compact a long-to-long mapping. Additionally, we’ll provide a detailed comparison table and discuss markup best practices.
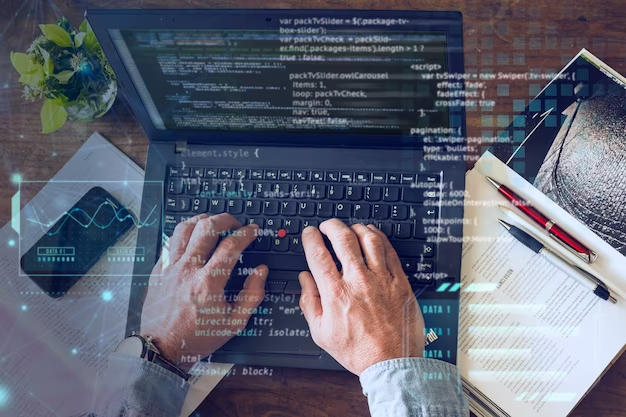
Understanding Java Long Long
In Java, the long data type is a 64-bit signed two’s complement integer. It can represent values ranging from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807. While long itself is a powerful data type, there are scenarios where you might need to create a mapping between two long values. This is where the concept of “Java Long Long” comes into play.
Use Cases for Java Long Long
- Efficiently Managing Large Datasets
When dealing with massive datasets, especially in fields like scientific research or big data analytics, mapping one long to another can be a memory-efficient way to handle data relationships. This approach minimizes memory consumption compared to using objects or complex data structures.
- High-Performance Indexing
In database systems and indexing engines, long-to-long mappings are used to optimize the retrieval of records. This is particularly beneficial when dealing with unique identifiers or primary keys in large databases.
- Cryptographic Applications
In cryptographic algorithms, where precision and security are paramount, long-to-long mappings can play a role in key generation and management.
Compact Long-to-Long Mapping: How-To
Creating a compact mapping between two long values involves using mathematical techniques to encode and decode the values efficiently. One common approach is to use bitwise operations, such as XOR, to map one long to another.
public class LongMappingExample { public static long encode(long valueToEncode, long mappingKey) { return valueToEncode ^ mappingKey; } public static long decode(long encodedValue, long mappingKey) { return encodedValue ^ mappingKey; } public static void main(String[] args) { long originalValue = 123456789; long mappingKey = 987654321; // Encode the value long encodedValue = encode(originalValue, mappingKey); // Decode the encoded value long decodedValue = decode(encodedValue, mappingKey); System.out.println(“Original Value: ” + originalValue); System.out.println(“Encoded Value: ” + encodedValue); System.out.println(“Decoded Value: ” + decodedValue); }} |
In this example, we use the XOR bitwise operator to create a mapping between originalValue and mappingKey. The encode function transforms the original value into an encoded form, while the decode function retrieves the original value from the encoded value using the same mapping key.
Comparison Table: Mapping Approaches
For a quick reference, let’s compare different approaches to long-to-long mapping:
Approach | Description | Use Cases |
---|---|---|
Bitwise Operations | Use XOR or other bitwise operations to create mappings | Efficient data compression, indexing, cryptography |
Hash Functions | Apply hash functions like SHA-256 for mapping | Data hashing, security |
Custom Algorithms | Create custom algorithms based on specific requirements | Tailored solutions |
Markup Best Practices
When discussing Java Long Long and its use cases on your website or in your documentation, consider the following markup best practices for improved SEO and readability:
1. Use Heading Tags
Structure your content with heading tags (<h1>, <h2>, etc.) to provide a clear hierarchy. For instance, use <h2> for section headings like “Use Cases for Java Long Long” and <h3> for subsections such as “Efficiently Managing Large Datasets.”
2. Add Code Blocks
When showcasing code examples, enclose them in <pre> or <code> tags to distinguish them from regular text. This makes it easier for readers to identify and understand the code snippets.
// Example of code within <pre> tagspublic static void main(String[] args) { // Your code here} |
3. Include Lists and Tables
Use HTML lists (<ul>, <ol>) for items like bullet points or numbered lists. Tables, as seen in the comparison table above, provide a structured way to present data.
<ul> <li>Item 1</li> <li>Item 2</li> <li>Item 3</li></ul> |
Conclusion
In this comprehensive exploration of “Java Long Long,” we’ve delved into the world of 64-bit integer mapping and its practical applications. Understanding how to efficiently map one long value to another can significantly enhance your ability to manage large datasets, optimize database indexing, and even strengthen the security of cryptographic systems.
We’ve learned that Java Long Long offers a memory-efficient approach to data mapping, which is particularly valuable in scenarios where resource utilization is critical. By harnessing mathematical techniques like bitwise XOR, you can create compact mappings that preserve data integrity and minimize memory overhead.
In addition to the practical coding examples and insights provided, we’ve discussed the importance of markup best practices to make your content both SEO-friendly and reader-friendly. By structuring your content with headings, using code blocks for code snippets, and incorporating tables for data comparison, you can present your information in a clear and organized manner.
FAQ
Java Long Long refers to the concept of efficiently mapping one long value to another, typically using mathematical techniques like bitwise operations. This approach is valuable in scenarios where memory optimization and data mapping are essential.
To improve the SEO-friendliness of your content, consider using proper markup, including heading tags (<h1>, <h2>), code blocks for code examples, and tables for data comparison. This helps search engines understand your content’s structure and enhances readability for your audience.
You can apply Java Long Long in various real-world scenarios, including scientific research, big data analytics, database management, and cryptographic applications. It offers a versatile approach to efficient data mapping that can benefit diverse fields and industries.
Java Long Long is used for:
1. Efficiently managing large datasets with minimal memory consumption;
2. Optimizing database indexing for improved query performance;
3. Enhancing security in cryptographic applications through precise data mapping.