In the world of Java programming, optimizing memory usage is often a critical concern. Java’s Singleton List is a powerful tool that allows you to create a list containing a single element, conserving memory when you need to work with singletons. In this article, we’ll explore the concept of a Java Singleton List, delve into memory-saving techniques specific to Java, present a detailed comparison table, and discuss best markup practices to make your code and explanations more accessible.
Understanding the Singleton List in Java
What is a Singleton List?
A Singleton List is a list that contains a single element. It’s a specialized use case of a list, particularly useful when you have a single item to store, retrieve, or manipulate. In Java, you can create a Singleton List using the Collections.singletonList() method. Here’s a simple example:
import java.util.Collections;import java.util.List; public class SingletonListExample { public static void main(String[] args) { String singleItem = “Hello, Singleton List!”; List<String> singletonList = Collections.singletonList(singleItem); // Accessing the single item String item = singletonList.get(0); System.out.println(item); }} |
In this code, we create a Singleton List containing a single string item. The Collections.singletonList() method ensures that the list contains only that specific element.
A Few More Memory-Saving Techniques in Java
While Singleton Lists are helpful for conserving memory when dealing with single items, there are several additional memory-saving techniques in Java that you should be aware of:
- Flyweight Design Pattern
The Flyweight design pattern is a structural pattern that allows you to share objects to reduce memory usage. It’s particularly useful when you have a large number of similar objects with shared characteristics. By separating intrinsic and extrinsic properties, you can create lightweight objects and reuse them efficiently.
- Object Pooling
Object pooling involves creating a pool of reusable objects and managing their lifecycle. Instead of constantly creating and destroying objects, you can acquire objects from the pool when needed and return them after use. This technique reduces the overhead of object creation and garbage collection.
- Weak References
Weak references in Java allow objects to be garbage-collected when there are no strong references to them. This can be beneficial when you need to hold references to objects but don’t want to prevent them from being collected when no longer needed. WeakHashMap is an example of using weak references for memory optimization.
- Immutable Objects
Immutable objects, once created, cannot be modified. They are thread-safe and can be safely shared among multiple threads. By using immutable objects, you reduce the risk of unintended changes and eliminate the need for synchronization, resulting in more efficient memory usage.
Comparison: Singleton List vs. ArrayList
Let’s compare a Singleton List to a regular ArrayList to understand when to use each:
Aspect | Singleton List | ArrayList |
---|---|---|
Memory Efficiency | More memory-efficient for single items | Less memory-efficient for single items |
Use Cases | Single-item storage and retrieval | Multiple-item storage and manipulation |
Performance | Faster for single-item operations | Faster for multi-item operations |
Memory Overhead | Minimal overhead for single items | Overhead increases with more items |
Mutability | Immutable (cannot add or remove items) | Mutable (items can be added or removed) |
Effective Markup Practices
To enhance the readability and accessibility of your Java code and explanations, consider the following markup practices:
Use of Headings
Organize your content with heading tags (<h1>, <h2>, etc.). For instance, use <h2> for section headings like “Understanding the Singleton List in Java” and <h3> for subsections such as “What is a Singleton List?”
Code Blocks
Enclose code examples in <pre> or <code> tags to distinguish them from regular text. This makes it easier for readers to identify and understand code snippets.
// Example of code within <pre> tagspublic static void main(String[] args) { // Your code here} |
Lists and Tables
Utilize HTML lists (<ul>, <ol>) for items like bullet points or numbered lists. Tables, as seen in the comparison table above, provide a structured way to present data.
<ul> <li>Item 1</li> <li>Item 2</li> <li>Item 3</li></ul> |
With a solid understanding of the Java Singleton List and additional memory-saving techniques in Java, you can optimize your code and reduce memory overhead effectively. Whether you’re working with singletons, implementing the Flyweight pattern, or exploring object pooling, these techniques will help you make your Java applications more memory-efficient and performant. By following the best markup practices, you ensure that your code and explanations are clear and accessible to your audience.
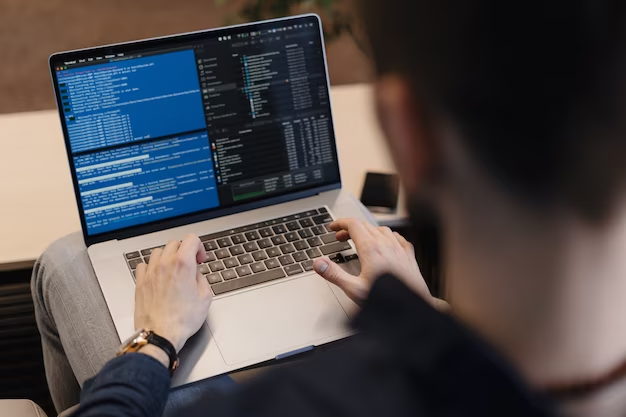
Conclusion
In the realm of Java programming, memory optimization is a paramount concern, and the Java Singleton List is a valuable tool in the arsenal of techniques to achieve it. By understanding how to create and utilize a Singleton List, you can efficiently store and manipulate single items, reducing memory overhead.
However, memory optimization in Java goes beyond just using Singleton Lists. It encompasses various techniques like the Flyweight design pattern, object pooling, weak references, and immutable objects. These techniques offer versatile solutions to different memory-related challenges, allowing you to build more efficient and performant applications.
As you navigate the vast landscape of Java programming, keep in mind that memory optimization is not a one-size-fits-all endeavor. It requires a deep understanding of your application’s requirements and careful consideration of the most suitable techniques.
FAQ
The primary advantage of using a Singleton List in Java is memory efficiency. It allows you to store a single item in a collection while avoiding the memory overhead associated with typical lists. This can be especially beneficial when you have singletons or unique elements to manage.
You should opt for a Singleton List when you specifically need to store and manipulate a single item. It’s a memory-efficient choice for scenarios where you want to avoid the overhead of creating a regular list with a single element.
No, you cannot add or remove elements from a Singleton List. Once created, it remains immutable, containing only the initially added element. If you need to modify the list, consider using a regular list instead.
Memory-saving techniques like the Flyweight pattern, object pooling, weak references, and immutable objects are valuable in various scenarios. They are commonly used in applications dealing with large datasets, multi-threading, or resource-constrained environments. For example, object pooling can help manage database connections efficiently, while the Flyweight pattern is useful in graphics rendering.