In the world of Java programming, optimizing the performance of your code is crucial for creating efficient and responsive applications. One area where performance gains can often be achieved is in the realm of Java collections. In this article, we will delve into the intricacies of Java Collections Performance. We will explore the fundamentals, common pitfalls, and strategies to enhance the efficiency of your Java collections. Let’s dive right in!
Java collections are a fundamental part of the Java programming language, providing a set of classes and interfaces to store, manage, and manipulate groups of objects. These collections are essential for tasks such as data storage, retrieval, and manipulation. However, like any software component, they come with performance considerations that developers must be aware of.
Types of Java Collections
Java offers a rich variety of collection types, each designed to serve specific purposes and address different requirements. These collections play a pivotal role in managing and organizing data in Java applications. Below, we delve into the four main types of Java collections: List, Set, Map, and Queue.
List
A List is an ordered collection that allows duplicate elements. It is typically used when you need to maintain elements in a specific order, such as maintaining a list of items in a shopping cart.
Common Implementations:
Implementation | Description |
---|---|
ArrayList | A dynamic array-based List that offers fast random access but can be slower when inserting or removing elements from the middle. |
LinkedList | A doubly-linked List that provides fast insertion and removal operations but slower random access compared to ArrayList. |
Use Cases:
- When order matters, and duplicates are allowed;
- Lists are ideal for scenarios like maintaining a history of user actions, managing a collection of items, or implementing a stack or queue.
Set
A Set is an unordered collection that does not allow duplicate elements. It is used when you want to store a collection of unique values without any particular order.
Common Implementations:
Implementation | Description |
---|---|
HashSet | A Set implemented using a hash table, which provides fast insertion and retrieval of elements. However, it does not guarantee any specific order. |
TreeSet | A Set implemented using a Red-Black tree, which offers elements in a sorted order. This implementation is slower for insertion and retrieval compared to HashSet. |
Use Cases:
- When you need to maintain a collection of unique values;
- Sets are suitable for implementing data structures like a set of unique user IDs or a dictionary with unique words.
Map
A Map is a collection that stores key-value pairs, where each key maps to a unique value. It is used when you need to associate values with specific keys for efficient retrieval.
Common Implementations:
Implementation | Description |
---|---|
HashMap | A Map implemented using a hash table. It provides fast key-value pair retrieval but does not guarantee any specific order of elements. |
TreeMap | A Map implemented using a Red-Black tree. It maintains elements in sorted order based on the keys. Retrieval is slower compared to HashMap. |
Use Cases:
- When you want to maintain a mapping between keys and values;
- Maps are commonly used in applications for caching data, looking up configuration settings, and implementing associative arrays.
Queue
Description: A Queue is a collection designed for specific insertion and removal patterns. It follows the FIFO (First-In-First-Out) order, meaning the first element added is the first to be removed.
Common Implementations:
Implementation | Description |
---|---|
PriorityQueue | A Queue implemented using a priority heap. Elements are removed in order of their priority. |
LinkedList | A doubly-linked list can also be used as a Queue by adding elements to the end and removing them from the front. |
Use Cases:
- When you need to manage elements based on their order of arrival or priority;
- Queues are vital for scheduling tasks, managing requests in web applications, and implementing algorithms like Breadth-First Search.
Performance Considerations
Time Complexity
One of the most critical aspects of Java collections performance is understanding the time complexity of various operations. Time complexity describes how the performance of an operation scales with the size of the collection. It is typically expressed using Big O notation.
Here’s a quick overview of the average time complexities for common collection operations:
Data Structure | Operation | Time Complexity |
---|---|---|
List | Access by index | O(1) |
Insertion/Removal | O(n) at the beginning | |
O(1) at the end | ||
O(n) in the middle | ||
Set | Addition (add) | O(1) on average (hash-dependent) |
Search (contains) | O(1) on average (hash-dependent) | |
Removal (remove) | O(1) on average (hash-dependent) | |
Map | Addition (put) | O(1) on average (hash-dependent) |
Search by key | O(1) on average (hash-dependent) | |
Removal by key | O(1) on average (hash-dependent) | |
Queue | Insertion (offer) | O(log n) for priority queues |
O(1) for other queues | ||
Removal (poll) | O(log n) for priority queues | |
O(1) for other queues |
Understanding these complexities is crucial for choosing the right collection type for your specific use case. For example, if you frequently need to perform operations that involve inserting or removing elements at the beginning of a list, LinkedList might be a better choice than ArrayList.
Choosing the Right Collection
Selecting the appropriate collection type for your needs is a key step in optimizing Java collections performance. Here are some guidelines to help you make the right choice:
- Use ArrayList for Random Access: If you need fast random access to elements and the list size doesn’t change frequently, ArrayList is a good choice due to its O(1) access time;
- Consider HashSet for Uniqueness: If you need to store a set of unique elements, HashSet provides O(1) average time complexity for add, remove, and contains operations;
- Use TreeMap for Sorted Maps: When you require a map with keys sorted in natural order, TreeMap offers efficient operations with O(log n) complexity;
- Use LinkedHashMap for Ordered Maps: If you need a map with predictable iteration order based on insertion order, LinkedHashMap is a suitable choice;
- Opt for PriorityQueues for Priority-Based Operations: When you need to prioritize elements, PriorityQueue provides efficient O(log n) insertion and removal for the highest-priority element.
Common Pitfalls
To achieve optimal Java collections performance, it’s crucial to avoid common pitfalls that can lead to inefficiencies in your code. Here are some pitfalls to watch out for:
- Excessive Copying: Creating unnecessary copies of collections can be a performance bottleneck. Be mindful of when and why you duplicate collections;
- Inefficient Iteration: Inefficiently iterating over collections using loops can lead to poor performance. Consider using enhanced for loops or Java Streams for more efficient iteration;
- Incorrect Synchronization: Using unsynchronized collections in multithreaded environments can result in data corruption or race conditions. Utilize synchronized collections or explicit synchronization as needed;
- Choosing the Wrong Collection: As mentioned earlier, selecting the wrong collection type for your use case can lead to poor performance. Always analyze your requirements before choosing a collection;
- Unchecked Casting: Avoid unchecked casting when working with collections to prevent runtime errors. Use parameterized types (generics) to ensure type safety.
Strategies for Enhanced Performance
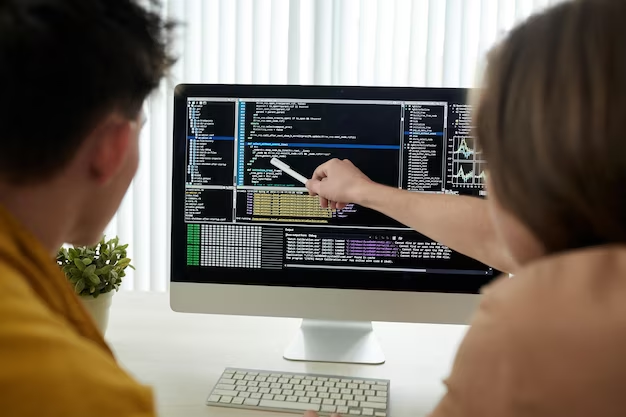
Now that we’ve covered the fundamentals and common pitfalls, let’s explore strategies to boost Java Collections Performance.
Utilize Generics
Generics are a powerful feature in Java that can significantly improve both code safety and performance. By specifying the types of objects a collection can hold, you gain compile-time type safety, reducing the likelihood of runtime errors. Moreover, generics enhance code readability. For instance:
List<String> stringList = new ArrayList<>(); stringList.add(“Java”); stringList.add(“Collections”); |
Initialize Collections with Proper Capacity
When creating collections, especially ArrayList, consider specifying an initial capacity. This helps avoid frequent resizing of the underlying array as elements are added, leading to performance gains, especially with large datasets:
List<Integer> numbers = new ArrayList<>(10000); |
Minimize Data Copying
Copying data between collections can be resource-intensive and detrimental to performance. Instead of manually copying elements one by one, use bulk operations like addAll and removeAll:
List<Integer> sourceList = new ArrayList<>(); List<Integer> targetList = new ArrayList<>(); // Inefficient copy for (Integer num : sourceList) { targetList.add(num); } // Efficient copy using addAll targetList.addAll(sourceList); |
Prefer Interface Types
Programming to interfaces rather than concrete implementations enhances flexibility. For instance, use List instead of specifying ArrayList in method signatures. This allows for easier switching between different collection types without altering method implementations:
public void processList(List<String> items) { // … } |
Implement Custom Comparators
In cases where you’re using collections like TreeSet and TreeMap, implementing custom comparators enables you to define custom ordering criteria for elements. This is especially valuable when sorting complex objects:
TreeSet<Student> students = new TreeSet<>(new StudentComparator()); |
Harness Java Streams
Java Streams provide a concise and efficient means of processing collections, promoting clean and readable code. They facilitate operations like filtering, mapping, and reduction without the need for explicit loops:
List<String> names = Arrays.asList(“Alice”, “Bob”, “Charlie”); // Using Java Streams to filter and print names names.stream() .filter(name -> name.length() > 3) .forEach(System.out::println); |
Employ Immutable Collections
In scenarios where data rarely changes, employing immutable collections can enhance both safety and performance. Libraries like Google Guava or Java 9’s List.of() provide immutable collections that eliminate the need for synchronization and improve thread safety:
List<String> immutableList = List.of(“Java”, “Collections”); |
Optimize for Multithreading
For applications involving multithreading, consider utilizing concurrent collections from the java.util.concurrent package. These collections are explicitly designed for thread safety and can greatly enhance performance in multithreaded environments.
Conclusion
Optimizing Java Collections Performance is a critical aspect of developing efficient Java applications. By understanding the time complexities of collection operations, choosing the right collection types, avoiding common pitfalls, and employing performance-enhancing strategies, you can ensure that your Java code performs at its best. Keep these principles in mind as you work with Java collections to create high-performance applications that meet your users’ needs.
FAQs
Generics provide type safety and prevent runtime errors by allowing you to specify the types of objects that a collection can hold. They also improve code readability and maintainability.
You can specify an initial capacity when creating an ArrayList to avoid frequent resizing. This can significantly improve performance for large datasets.
Yes, there are cases where copying data is necessary, such as when you need to create a defensive copy to protect the original data from modification. However, you should minimize unnecessary copying to improve performance.
Concurrent collections from the java.util.concurrent package should be used when your application involves multiple threads accessing and modifying collections simultaneously. They provide thread safety and can enhance performance in multithreaded environments.
Java Streams provide a concise and efficient way to process collections. They allow you to express complex operations on collections in a more readable and declarative manner, often leading to improved performance and maintainability.