In the vast world of programming, data integrity and error-checking are paramount. This is where CRC32 and Adler32, two widely-used checksum algorithms in Java, step in to ensure the integrity of data transmission. In this post, we’ll delve deep into the world of CRC32 and Adler32 in Java. By the end of this comprehensive guide, you’ll not only understand how to implement these checksum algorithms but also their significance in various applications.
CRC32
What is CRC32?
CRC32, short for Cyclic Redundancy Check, is a widely used checksum algorithm in the world of computing. It plays a crucial role in ensuring data integrity during transmission or storage. In essence, CRC32 generates a fixed-size checksum (usually 32 bits) based on the content of the data. This checksum is appended to the data, allowing the receiver to verify if the data has been altered in any way.
How CRC32 works?
CRC32 operates by treating the data as a sequence of bits and performing polynomial division on these bits. The result of this division is the CRC32 checksum. When the data is received, the same polynomial division is applied to the received bits. If the result matches the transmitted CRC32 checksum, the data is considered intact; otherwise, it may have been corrupted.
- CRC32, which stands for Cyclic Redundancy Check, operates on a stream of data, treating it as a sequence of bits;
- It uses polynomial division to calculate a fixed-size checksum, typically 32 bits in length;
- The data is treated as a binary polynomial, and the CRC32 algorithm applies polynomial division to generate the checksum;
- A predefined polynomial, known as the “generator polynomial,” is used in the division process;
- The polynomial division involves bitwise XOR operations and shifting;
- The result of the division is the CRC32 checksum, a fixed-size value that represents the integrity of the data;
- When data is transmitted or stored, the CRC32 checksum is often appended to it;
- Upon receiving the data, the same CRC32 algorithm is applied to the received data;
- If the calculated CRC32 checksum matches the received checksum, the data is considered intact; otherwise, it may have been corrupted during transmission;
- The strength of CRC32 lies in its ability to detect a wide range of errors efficiently, making it suitable for applications where data integrity is critical, such as network communication and data storage.
Use cases of CRC32 in Java
CRC32 finds applications in various domains, including network communication, data storage, and error detection in files. In Java, you can use the java.util.zip.CRC32 class to calculate CRC32 checksums efficiently.
Implementation of CRC32 in Java
Here’s a simple example of how to calculate a CRC32 checksum in Java:
import java.util.zip.CRC32;
public class CRC32Example {
public static void main(String[] args) {
CRC32 crc32 = new CRC32();
String data = "Hello, CRC32!";
byte[] bytes = data.getBytes();
crc32.update(bytes);
long checksum = crc32.getValue();
System.out.println("CRC32 Checksum: " + checksum);
}
}
In this code, we create an instance of CRC32, update it with the data, and obtain the checksum value.
Adler32
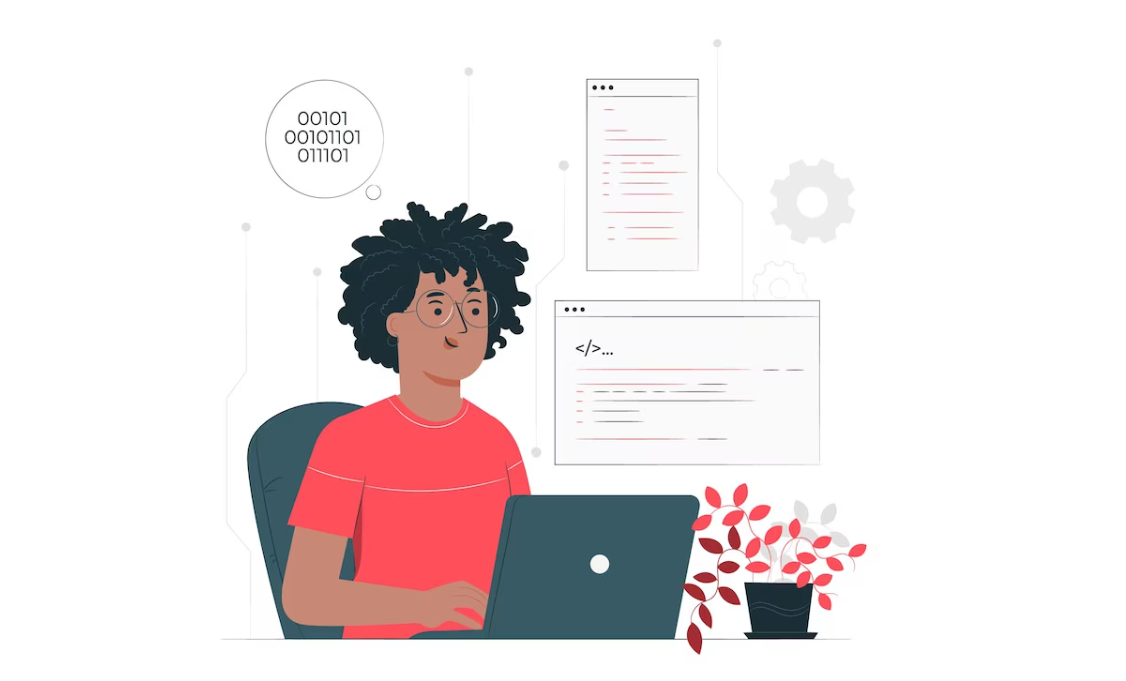
What is Adler32?
Adler32, often regarded as a robust checksum algorithm, plays a vital role in ensuring the integrity of data across various computing applications. Unlike CRC32, it offers a simpler and faster method for verifying data integrity by generating a checksum based on the cumulative sum of data bytes, employing a rolling sum technique that factors in the byte order. Its speed and efficiency make it particularly well-suited for real-time applications and small to medium-sized data blocks. However, it’s essential to acknowledge that Adler32 may exhibit limitations in situations where stringent error detection is required or when dealing with larger datasets or less reliable data transmission channels. In such cases, the more comprehensive error-checking capabilities of CRC32 are often preferred.
How Adler32 works?
Adler32 calculates a checksum by summing the values of all bytes in the data, along with a rolling sum of these values. This rolling sum ensures that the order of bytes affects the final checksum, providing a higher level of error detection.
- Adler32 operates on a stream of data, treating it as a sequence of bytes.
- It maintains two 16-bit checksum values, A and B, initially set to 1.
- Adler32 processes each byte in the data, updating A and B in a rolling fashion.
- A is updated by adding the byte value to its current value modulo 65521.
- B is updated by adding the current value of A to its own value modulo 65521.
- The final Adler32 checksum is obtained by combining A and B, with B shifted left by 16 bits and then added to A.
- The result is a 32-bit checksum that reflects the cumulative effect of all bytes in the data.
- This rolling sum approach ensures that both the content and the order of bytes contribute to the checksum, enhancing error detection capabilities.
Comparing Adler32 and CRC32
Adler32 is faster and more efficient for short data blocks, while CRC32 is often preferred for longer data streams due to its superior error-detection capabilities.
Aspect | Adler32 | CRC32 |
---|---|---|
Algorithm Complexity | Simpler and faster, suitable for short data blocks | More complex, suitable for longer data streams |
Error Detection | Good for detecting errors but less robust than CRC32 | Highly robust, can detect a wider range of errors |
Speed | Faster, ideal for applications requiring high-speed checksums | Slower compared to Adler32 for short data but efficient for long data streams |
Checksum Length | Generates a 32-bit checksum | Generates a 32-bit checksum |
Checksum Uniqueness | Possibility of collisions in rare cases | Extremely low collision probability |
Memory Usage | Requires minimal memory | Requires more memory for calculations |
Use Cases | Ideal for small data blocks or real-time applications | Preferred for longer data transmissions, storage, and critical applications |
Java Implementation | Utilizes java.util.zip.Adler32 class | Utilizes java.util.zip.CRC32 class |
Use cases of Adler32 in Java
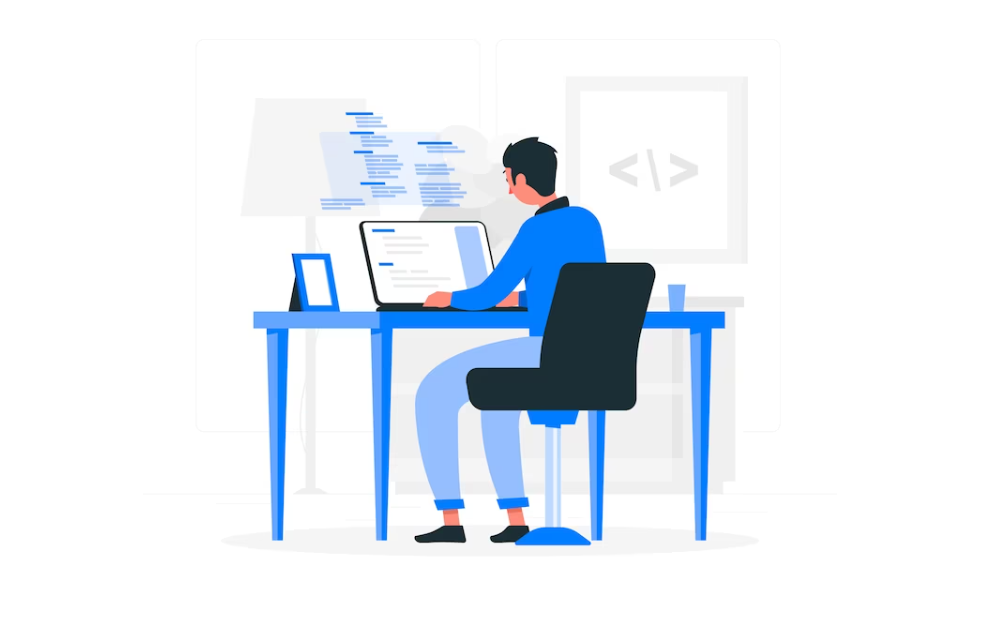
Adler32 is commonly used in applications where speed is a priority, such as in checksumming small data packets or files.
Implementation of Adler32 in Java
Here’s an example of how to calculate an Adler32 checksum in Java:
import java.util.zip.Adler32;
public class Adler32Example {
public static void main(String[] args) {
Adler32 adler32 = new Adler32();
String data = "Hello, Adler32!";
byte[] bytes = data.getBytes();
adler32.update(bytes);
long checksum = adler32.getValue();
System.out.println("Adler32 Checksum: " + checksum);
}
}
In this code, we use the java.util.zip.Adler32 class to calculate the Adler32 checksum for the given data.
Conclusion
This comprehensive guide explores Java CRC32 and Adler32 checksum algorithms, vital for data integrity and error-checking. CRC32 safeguards data during transmission or storage, generating a 32-bit checksum through polynomial division. Adler32, a faster alternative, uses rolling sum for checksum calculation. While Adler32 suits real-time and smaller data, CRC32 excels in detecting various errors, ideal for longer transmissions and critical applications. Gain insights into implementing these algorithms and understand their significance in Java programming’s evolving data integrity and reliability.