Java, a versatile and widely used programming language, provides a rich set of data types to handle various kinds of information. In this comprehensive guide, we will delve deep into Java’s primitive data types: byte, short, int, long, char, and explore the fascinating world of boxing and unboxing. Understanding these fundamental concepts is essential for writing efficient and robust Java code.
Java Primitive Data Types
Java Primitive Data Types form the bedrock of data storage and manipulation within the Java programming language. These data types are the fundamental building blocks for representing and working with basic values, such as numbers and characters. The roster includes integers (like byte, short, int, and long) for handling whole numbers, floating-point types (such as float and double) for decimal values, the char type for individual characters, and boolean for true or false values.
One defining feature of primitive data types is their simplicity and efficiency. Unlike objects, they are not associated with methods or properties and are stored directly in memory. This direct representation makes them highly efficient, particularly in situations where performance optimization is critical.
For Java developers, a solid grasp of these primitive data types is indispensable. They serve as the building blocks upon which more complex data structures and objects are constructed, making them essential knowledge for crafting efficient and effective Java applications. Understanding the nuances of these data types is a fundamental step towards becoming a proficient Java programmer.
byte:
The byte data type is the smallest integer type in Java, occupying just 8 bits (1 byte). Despite its size, it packs quite a punch when it comes to memory efficiency. It can hold values ranging from -128 to 127. Developers often use byte for tasks where every bit of memory optimization counts, such as in embedded systems, file I/O, and image processing.
- Example: In an embedded systems application, you might use the byte data type to store sensor readings where memory optimization is crucial. For instance, representing temperature values ranging from -50°C to 50°C can be efficiently handled using byte.
short:
The short data type is a 16-bit integer, allowing values in the range of -32,768 to 32,767. Like byte, it’s used to conserve memory, particularly when dealing with integers within this range. You might encounter it in scenarios like handling pixel values in images or storing small counters efficiently.
- Example: Imagine you’re developing a graphics processing application. The short data type could be employed to store pixel values, where each pixel’s color intensity ranges from 0 to 255. Using short conserves memory while accommodating the required range of values.
int:
When it comes to whole numbers, the int data type is the workhorse of Java. It’s a 32-bit integer that can accommodate values from -2^31 to 2^31-1. In most Java applications, you’ll find int used extensively for variables like loop counters, array indices, and many other everyday tasks.
- Example: In a simple loop that iterates through an array of integers to find the sum, the loop counter is typically declared as an int. For instance, for(int i = 0; i < array.length; i++) where i is an int, efficiently handles array indexing.
long:
For situations requiring large integers, the long data type comes to the rescue. It’s a 64-bit integer, capable of holding values ranging from -2^63 to 2^63-1. long is commonly used in scientific calculations, financial applications, and any scenario where precision with big numbers is crucial.
- Example: In financial applications, you might need to store large numbers with precision. For instance, when calculating the national debt of a country, the long data type can handle the astronomical figures involved, ensuring accuracy.
char:
Unlike the other numeric types, char doesn’t deal with numbers. Instead, it represents characters. It’s a 16-bit data type capable of holding Unicode characters. This makes it suitable for internationalization and text processing, ensuring that Java can handle characters from various languages and scripts with ease.
- Example: Consider a multilingual text editor application. The char data type would be used to represent individual characters, allowing users to input and edit text in different languages, scripts, and character sets, thanks to its Unicode support.
Boxing and Unboxing
Process | Description | Example |
---|---|---|
Boxing | Converting a primitive data type into its corresponding wrapper class object. | int to Integer, char to Character |
Autoboxing | Automatic boxing in Java, simplifying code and enhancing readability. | int to Integer, char to Character |
Unboxing | Extracting the original value from a wrapper class object. | Integer to int, Character to char |
Autounboxing | Automatic unboxing in Java, seamlessly converting wrapper objects to their primitive counterparts. | Integer to int, Character to char |
Benefits of Boxing and Unboxing
Boxing and unboxing provide several advantages in Java development:
Collections and Generics
- Collections: Java collections like ArrayList and HashMap can only store objects, not primitive data types. Boxing allows you to use these collections with primitive values by converting them into objects when needed;
- Generics: Generics in Java work seamlessly with wrapper classes. This means you can create generic classes and methods that can handle both primitive data types and objects, providing flexibility and code reusability.
Nullable Values
- Nullable Values: Wrapper classes can represent null values, whereas primitive data types cannot. This is particularly useful when dealing with databases, APIs, or other scenarios where a value may or may not be present.
Enhanced Readability
- Enhanced Readability: Boxing and unboxing make your code more readable and concise. You can use primitive data types when performance is critical and leverage boxing when working with collections, generics, or nullable values without cumbersome conversions.
Converting long to int in Java
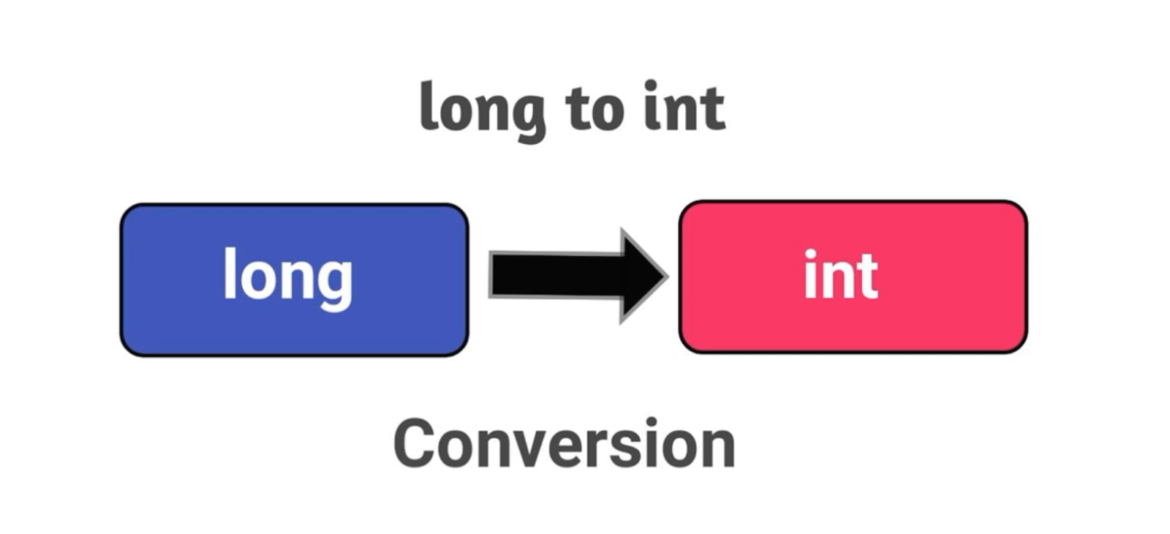
In Java, converting a long to an int is a common operation when you need to work with large numbers but want to preserve memory efficiency. The long data type is a 64-bit signed integer, capable of representing a wide range of values. On the other hand, the int data type is a 32-bit signed integer, which can represent a smaller range of values compared to long.
When performing a conversion from long to int, it’s important to be aware of potential data loss. If the long value falls within the valid range of int (from -2^31 to 2^31-1), the conversion can be done safely. However, if the long value exceeds this range, it may result in data truncation, and the result in the int variable will not accurately represent the original long value.
Java provides mechanisms for explicit casting from long to int, allowing you to perform this conversion while acknowledging the potential loss of data precision. Careful consideration of data range and potential data loss is crucial when converting between these two data types to ensure the integrity and accuracy of your Java program’s calculations.
Example 1: Converting a long within the int range
long longValue = 12345L;
int intValue = (int) longValue;
System.out.println("Original long value: " + longValue);
System.out.println("Converted int value: " + intValue);
Output:
Original long value: 12345
Converted int value: 12345
In this example, the long value 12345 is within the valid range of int, so the conversion results in no data loss, and the values remain the same.
Example 2: Converting a long exceeding the int range
long longValue = 2147483648L; // This value is outside the int range
int intValue = (int) longValue;
System.out.println("Original long value: " + longValue);
System.out.println("Converted int value: " + intValue);
Output:
Original long value: 2147483648
Converted int value: -2147483648
In this example, the long value 2147483648 exceeds the valid range of int, resulting in data truncation. The conversion causes an overflow, and the resulting int value is -2147483648.
These examples illustrate how converting a long to an int in Java can lead to data loss when the long value falls outside the valid range of the int data type. It’s essential to be cautious and consider potential data truncation when performing such conversions.
Use Cases and Best Practices
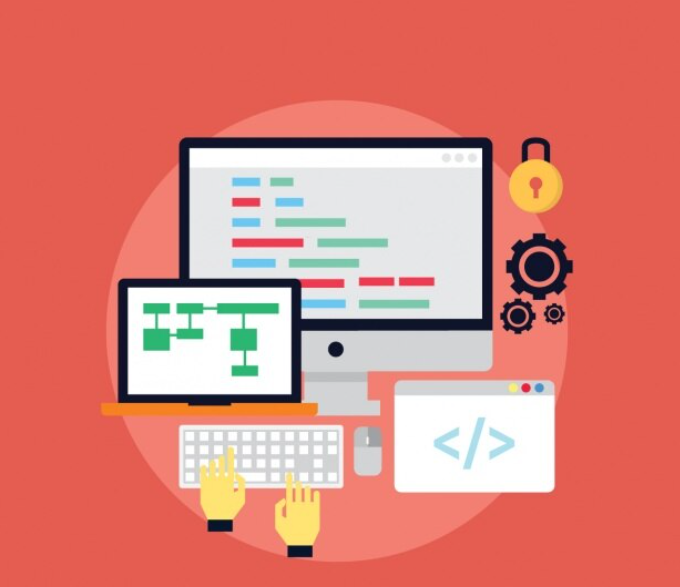
- Choosing the Right Data Type: When selecting between byte, short, int, and long, consider the range and precision required for your data. Choose smaller types like byte or short for memory optimization and larger types like int or long for larger numbers;
- Char for Characters: Use the char data type when working with characters, strings, or text. Remember that char represents individual characters, not strings. For strings, you would typically use the String class;
- Leveraging Boxing and Unboxing: Embrace boxing and unboxing when you need to work with collections, generics, or nullable values. It simplifies your code and makes it more readable;
- Performance Considerations: While boxing and unboxing provide convenience, they can introduce a small performance overhead due to the creation of wrapper objects. In performance-critical scenarios, be mindful of this and consider using the primitive data types directly.
Conclusion
In this comprehensive exploration of Java’s primitive data types and the concepts of boxing and unboxing, you’ve gained a deeper understanding of how Java handles different types of data. These fundamental concepts are the building blocks of efficient and robust Java programming. By choosing the right data types, leveraging boxing and unboxing strategically, and considering performance implications, you can write code that is both elegant and efficient. Mastering these core concepts is a crucial step toward becoming a proficient Java developer.