Logging is the process of recording information about an application’s runtime behavior. It plays a crucial role in debugging, error tracking, and performance monitoring. In the context of Java, effective logging ensures that you have the necessary insights to identify and resolve issues promptly.
The Role of Logging in Application Monitoring
Java logging is not just about capturing errors; it’s a vital tool for monitoring the health and performance of your application. By analyzing logs, you can:
- Track Application Behavior: Understand how your application behaves in different scenarios;
- Detect Anomalies: Spot unusual patterns or errors that could indicate issues;
- Measure Performance: Gather data on execution times and resource usage;
- Support Troubleshooting: Provide valuable information for diagnosing and resolving problems.
Challenges in Java Logging Performance
While logging is essential, it can impact your application’s performance if not managed correctly. Common challenges include:
- Excessive Logging: Logging too much information can overwhelm your system;
- Inefficient Logging Code: Poorly written log statements can slow down your application;
- Inadequate Configuration: Choosing inappropriate log levels or settings can lead to issues.
Java Logging Frameworks: A Comparative Analysis
Java offers several logging frameworks, each with its own set of features and characteristics. Let’s compare three popular options: Log4j, Logback, and java.util.logging (JUL).
Log4j vs. Logback vs. JUL
Log4j:
- Mature and widely used;
- Rich configuration options;
- Supports appenders and layouts for versatile log formatting.
Logback:
- Successor to Log4j;
- Improved performance;
- Designed with extensibility in mind.
JUL (java.util.logging):
- Included in Java SE;
- Simplicity and ease of use;
- Limited configurability compared to Log4j and Logback.
Pros and Cons of Each Framework
Log4j:
- Pros: Extensive community support, highly customizable;
- Cons: Slower than Logback in some scenarios.
Logback:
- Pros: Superior performance, pluggable architecture;
- Cons: Smaller user base compared to Log4j.
JUL (java.util.logging):
- Pros: Out-of-the-box integration, simplicity;
- Cons: Less flexible configuration options.
Choosing the Right Framework for Your Project
The choice of a logging framework depends on your project’s requirements and constraints. Consider factors like performance, configurability, and community support when making your decision.
Configuring Logging Levels for Efficiency
One of the fundamental aspects of Java logging is setting the right log levels. Log levels indicate the severity of log messages and help you control the amount of information generated by your application.
Exploring Log Levels (INFO, DEBUG, WARN, ERROR)
- INFO: General information about application execution;
- DEBUG: Detailed debugging information for troubleshooting;
- WARN: Non-critical issues that may require attention;
- ERROR: Critical errors that need immediate intervention.
Setting the Appropriate Log Level
Choosing the right log level is crucial to balance information and performance. Logging too much information can lead to performance bottlenecks, while logging too little can hinder troubleshooting.
Dynamic Logging Level Adjustments
Consider implementing dynamic log level adjustments to fine-tune logging in real-time. This allows you to increase logging for specific components during debugging and reduce it during normal operation.
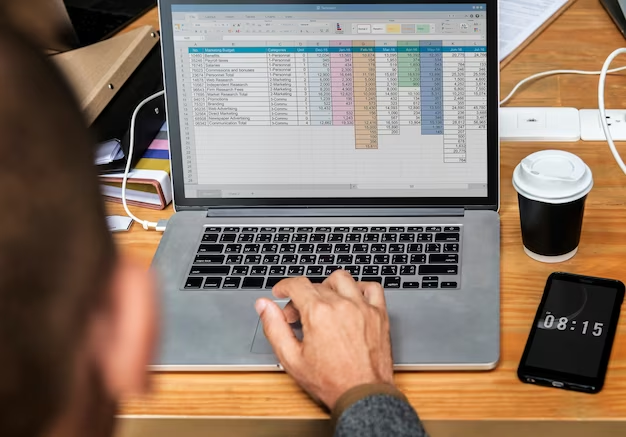
Analyzing Logging Overhead
Measuring Logging Overhead
To truly optimize Java logging performance, it’s crucial to understand the overhead it introduces to your application. Overhead refers to the additional computational resources and time consumed by logging operations.
To measure logging overhead, you can employ profiling tools and performance monitoring software. These tools provide insights into how much CPU, memory, and disk I/O your logging activities consume.
Keep in mind that the impact of logging overhead can vary depending on the logging framework, log levels, and the volume of log messages generated. Profiling helps identify bottlenecks and areas where optimization is most needed.
Profiling and Benchmarking Logging
Profiling is the process of gathering data on the runtime behavior of your application, including the performance of logging operations. Profiling tools like YourKit, VisualVM, or Java Mission Control allow you to:
- Identify the most frequently called log statements;
- Measure the execution time of log-related code;
- Discover memory usage patterns associated with logging.
Benchmarking involves comparing different logging configurations or frameworks to determine which one offers the best performance for your specific use case. Be sure to benchmark under realistic conditions that mimic your production environment.
Reducing Logging Overhead with Asynchronous Logging
One effective strategy to mitigate logging overhead is to implement asynchronous logging. Asynchronous logging offloads the actual logging work to separate threads or processes, allowing your application to continue running without interruption.
By using asynchronous logging, you can achieve the following benefits:
- Reduced impact on application responsiveness;
- Better utilization of multi-core processors;
- Improved overall system performance.
Popular logging frameworks like Logback and Log4j2 offer asynchronous logging options. However, be cautious when configuring asynchronous logging to avoid potential issues like log message reordering.
Log Analysis and Monitoring Tools
Elasticsearch, Logstash, and Kibana (ELK Stack)
The ELK Stack, consisting of Elasticsearch, Logstash, and Kibana, is a powerful combination of tools for log analysis and monitoring in distributed systems.
- Elasticsearch: A highly scalable search and analytics engine that stores and indexes log data for fast retrieval;
- Logstash: A data processing pipeline that ingests and processes log data from various sources, making it ready for analysis;
- Kibana: A user-friendly web interface that allows you to visualize log data, create custom dashboards, and set up alerts.
The ELK Stack is well-suited for large-scale applications and microservices architectures, providing real-time insights into your logs.
Splunk
Splunk is another robust log analysis and monitoring solution that offers powerful search and visualization capabilities. It excels at ingesting and indexing log data from diverse sources and provides advanced querying options.
Key features of Splunk include:
- Search and Reporting: Quickly locate and analyze log data using a rich query language;
- Dashboards: Create custom dashboards with real-time data visualizations;
- Alerting: Set up alerts to notify you of specific log events or anomalies;
- Machine Learning: Utilize machine learning for anomaly detection and predictive analysis.
Splunk is a commercial solution with a strong reputation in the industry, making it a popular choice for enterprises.
Custom Dashboards and Alerts
For more flexibility in log analysis and monitoring, consider building custom dashboards and alerts tailored to your application’s specific needs.
Tools like Grafana and Prometheus allow you to create highly customizable dashboards for visualizing log data. You can combine these tools with alerting systems like Alertmanager to receive notifications when log events meet predefined criteria.
Custom dashboards and alerts give you the freedom to design monitoring solutions that align perfectly with your application’s requirements, ensuring you stay informed about its health and performance.
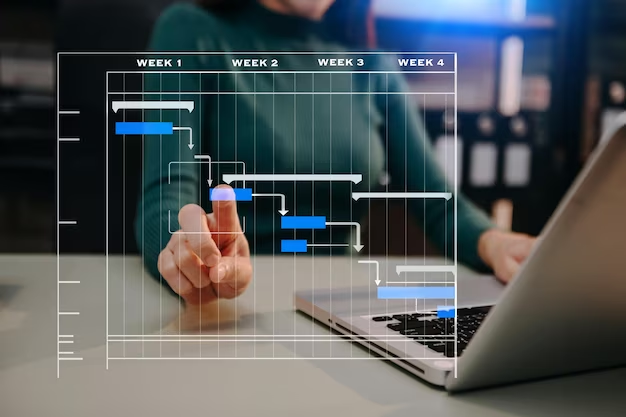
Advanced Logging Techniques
Log Rotation Strategies
Log rotation is a crucial aspect of maintaining efficient log files. It involves managing log files by creating new ones when a certain size or time threshold is reached. Here are some common log rotation strategies:
- Size-Based Rotation: Rotate log files when they reach a specific size (e.g., 10 MB). This prevents individual log files from growing too large and consuming excessive disk space;
- Time-Based Rotation: Rotate log files at regular intervals (e.g., daily or weekly). This ensures that log files remain manageable and organized;
- Combined Rotation: Implement a combination of size-based and time-based rotation to strike a balance between file size and log retention.
Avoiding Common Logging Pitfalls
Effective logging requires careful consideration to avoid common pitfalls that can degrade performance and usefulness. Here are some pitfalls to watch out for:
- Over-Logging: Excessive log messages can flood your log files and impact application performance. Be selective about what you log;
- Under-Logging: Failing to log critical information can make troubleshooting difficult. Ensure you capture essential details;
- Inefficient Logging Code: Poorly written log statements can introduce unnecessary overhead. Optimize your logging code for performance.
Logging Security Best Practices
Logging can expose sensitive information if not handled securely. To protect your application and its users, follow these security best practices:
- Avoid Logging Sensitive Data: Never log sensitive information like passwords or personal data. Implement proper filtering or redaction;
- Secure Log Files: Restrict access to log files to authorized personnel only. Encrypt log files if they contain sensitive data;
- Log Injection Prevention: Sanitize user-generated input to prevent log injection attacks that can manipulate log messages.
Exploring Real-World Java Logging Performance
In this section, we’ll examine real-world scenarios where optimizing Java logging performance is essential. We’ll explore case studies and success stories from notable companies and projects.
Case Study: Netflix
Netflix, a pioneer in online streaming, handles vast amounts of user data and system logs. They’ve invested heavily in optimizing their Java logging infrastructure. Key takeaways from Netflix’s approach include:
- Asynchronous Logging: Netflix employs asynchronous logging to minimize performance impact on their streaming platform;
- Dynamic Log Levels: They use dynamic log level adjustments to control log verbosity in real-time, allowing them to troubleshoot issues efficiently;
- Centralized Logging: Netflix relies on centralized logging and analytics tools to gain insights into their distributed microservices architecture.
Case Study: Twitter
Twitter, a social media giant, processes a colossal volume of tweets and interactions daily. Their Java logging practices focus on scalability and real-time insights. Notable aspects of Twitter’s logging strategy include:
- Efficient Log Analysis: Twitter employs custom log analysis tools to identify trends and anomalies in real-time, helping them respond swiftly to user issues;
- Log Correlation: Correlating log events across their vast infrastructure enables Twitter to pinpoint the source of problems quickl;
- Automated Alerts: Automated alerting mechanisms notify Twitter’s operations teams of critical log events, ensuring rapid response.
Emerging Trends in Java Logging
As technology evolves, so does the field of Java logging. Stay informed about emerging trends and tools that can further enhance your logging performance:
Cloud-Native Logging
With the rise of cloud-native applications, logging has become more complex. Tools like Fluentd, Fluent Bit, and Loki are gaining popularity for their ability to handle log aggregation and routing in cloud-native environments.
Observability and Distributed Tracing
Observability solutions like OpenTelemetry and Jaeger enable in-depth analysis of distributed systems. These tools provide insights beyond traditional logs and help diagnose complex issues in microservices architectures.
Machine Learning-Powered Log Analysis
Machine learning algorithms are increasingly used to analyze logs and detect anomalies automatically. Tools like Anomaly Detective and LogRocket use AI to identify unusual behavior in log data.
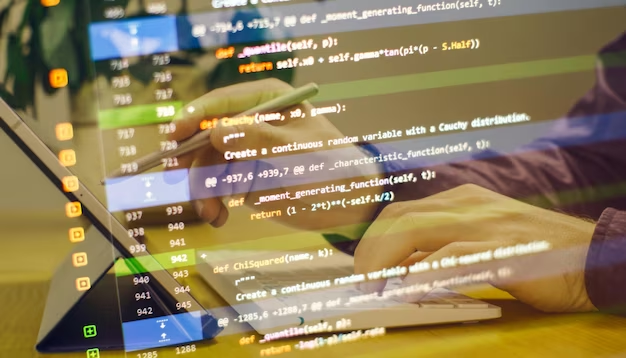
Conclusion
In this comprehensive guide, we’ve explored the world of Java logging performance. You’ve learned about different logging frameworks, configuring log levels, optimizing log file output, and managing logging in distributed systems. By implementing the best practices discussed here, you can achieve peak Java logging performance and ensure your applications run smoothly.
FAQs
Logging in Java applications serves the purpose of recording runtime behavior, facilitating debugging, and monitoring performance.
The choice depends on factors like performance requirements, configurability, and community support. Consider options like Log4j, Logback, and JUL.
Common challenges include excessive logging, inefficient logging code, and inadequate configuration, all of which can impact application performance.
Setting the right log level helps balance the amount of information generated by your application, preventing both information overload and inadequate troubleshooting data.
Optimizing log message formatting involves crafting efficient log messages that provide valuable information without adding unnecessary complexity to the log output.