Java, a renowned programming language celebrated for its versatility, provides developers with a myriad of tools to craft efficient and readable code. One such tool is varargs, short for “variable-length argument lists,” which empowers developers to pass a variable number of arguments to a method with ease. This feature, while offering undeniable flexibility, harbors hidden performance implications that often go unnoticed.
What are varargs?
Varargs, short for “variable-length arguments,” are a feature in the Java programming language that provides a way to pass a variable number of arguments to a method. Essentially, they allow you to create methods that can accept an arbitrary number of parameters of the same type, making your code more flexible and adaptable. This means you can call a method with different numbers of arguments without having to define multiple overloaded versions of that method. Varargs are indicated in Java by an ellipsis (…) followed by the data type of the arguments in the method’s parameter list.
How are varargs declared and used in Java?
Declaring and using varargs in Java involves defining a method with varargs as a parameter. To declare varargs, you add an ellipsis (…) followed by the data type of the arguments you expect to receive in the method’s parameter list. When calling the method, you can pass any number of arguments of the specified data type separated by commas. Java internally converts these arguments into an array, making it easier for you to work with them within the method. This feature simplifies method calls when the number of arguments varies, enhancing code readability and reducing the need for multiple method overloads.
Why are varargs popular among developers?
Varargs have gained popularity among developers for several compelling reasons.
- First and foremost, they offer a significant degree of convenience and flexibility when designing methods that accept variable numbers of arguments;
- This simplifies the API of methods, making them easier to use and understand;
- Additionally, varargs can reduce code redundancy by eliminating the need to create multiple overloaded methods to accommodate varying argument counts;
- This flexibility, combined with improved code readability, makes varargs a valuable tool in Java development, especially in scenarios where you want to design user-friendly and adaptable APIs.
The Performance Trade-off
When it comes to using varargs in Java, there is a nuanced performance trade-off that developers should be aware of. While varargs provide the convenience of passing a variable number of arguments to a method, they introduce certain hidden costs that can impact the efficiency of your code. These costs primarily stem from the dynamic nature of varargs, which requires Java to perform additional operations behind the scenes, ultimately affecting the execution speed and memory usage of your program. Therefore, understanding this performance trade-off is crucial for making informed decisions about when and how to use varargs effectively.
The Hidden Cost of Varargs
The hidden cost of varargs becomes apparent when you consider the extra work Java has to do to accommodate variable argument lists. Each time a method with varargs is invoked, Java must create an array to hold the arguments you passed in. This array creation incurs both time and memory overhead. The dynamic nature of varargs also means that additional runtime checks are necessary to ensure the correct number of arguments has been provided, further contributing to the cost. While these overheads may be negligible in many scenarios, they can accumulate in performance-critical applications, potentially leading to bottlenecks that affect your program’s responsiveness.
How Varargs Affect Method Invocation
Varargs influence method invocation in a way that differs from methods with fixed argument lists. When you call a method that accepts varargs, Java must determine how many arguments you’ve provided and then package them into an array. This added step in the method invocation process introduces a minor but measurable overhead compared to methods with a fixed number of arguments. Additionally, the flexibility of varargs can lead to ambiguity in method resolution when overloaded methods are present, requiring Java to perform extra work to identify the correct method to invoke. Therefore, understanding how varargs affect method invocation is essential for optimizing your code and minimizing performance bottlenecks.
Memory Overhead and Its Implications
Memory overhead is a critical aspect of varargs that has far-reaching implications for your Java programs. When you use varargs, Java dynamically creates an array to store the provided arguments, and this array consumes additional memory. In scenarios where methods with varargs are frequently called, this can lead to increased memory usage, potentially impacting the overall memory footprint of your application. Consequently, it’s essential to consider the memory implications of varargs, especially in resource-constrained environments or applications where memory optimization is crucial. Careful management of memory overhead is key to maintaining the efficiency and scalability of your Java code.
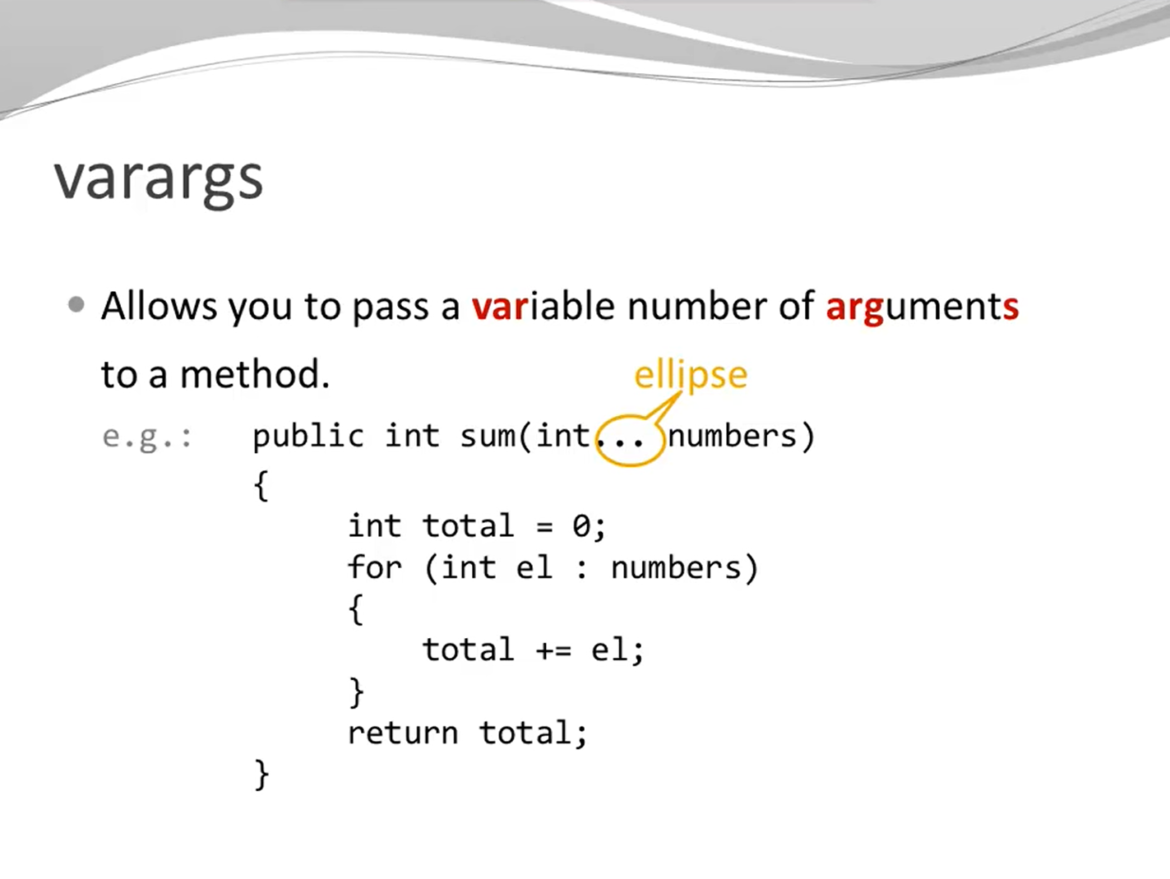
Profiling Your Code
When it comes to optimizing code that involves Java varargs, it’s essential to employ the right tools for performance profiling. Profiling is the process of analyzing your application’s execution to identify performance bottlenecks and areas that could benefit from optimization. Profiling tools provide valuable insights into your code’s behavior, helping you make informed decisions about where to focus your optimization efforts.
- Tools for Performance Profiling: A wide range of performance profiling tools are available to Java developers, each offering unique features and capabilities. These tools enable you to gather data on various aspects of your application, such as CPU usage, memory usage, and execution time. Some popular profiling tools include Java VisualVM, YourKit, and Visual Studio Profiler. By utilizing these tools, you can gain a comprehensive understanding of how your code performs in real-world scenarios;
- Identifying Varargs-Related Bottlenecks: In the context of varargs, performance profiling becomes particularly valuable. Profiling tools can help you pinpoint varargs-related bottlenecks within your codebase. These bottlenecks may include excessive memory allocation due to varargs array creation or performance degradation caused by frequent method invocations involving varargs. Profiling allows you to identify precisely where these issues occur, enabling you to prioritize optimization efforts effectively;
- Real-World Examples of Performance Issues: To illustrate the importance of performance profiling and optimization in the presence of Java varargs, it’s helpful to examine real-world examples of performance issues. These examples provide concrete scenarios where varargs-related bottlenecks were detected and addressed. By studying these cases, you can gain insights into common pitfalls and learn how to apply optimization techniques effectively in your own projects.
Optimization Techniques
- Limiting Varargs Usage: Be selective in using varargs. While they offer flexibility, weigh their benefits against performance costs. Reserve varargs for scenarios demanding dynamic arguments, reducing unnecessary overhead;
- Switching to Fixed Arguments: For consistent argument counts, switch to fixed-length lists. They eliminate varargs’ dynamic array creation, reducing memory and processing overhead, enhancing predictability, and maintaining code quality;
- Leveraging Method Overloading: Improve code efficiency by exploiting method overloading when handling variable arguments. Overloaded methods with varying parameters can eliminate array creation, enhancing execution and method resolution performance;
- Array Parameters Instead of Varargs: Instead of varargs, use explicit array parameters for variable-length arguments. This approach grants better memory control and efficient array manipulation, particularly valuable for memory-critical scenarios.
When to Use Varargs and When to Avoid Them
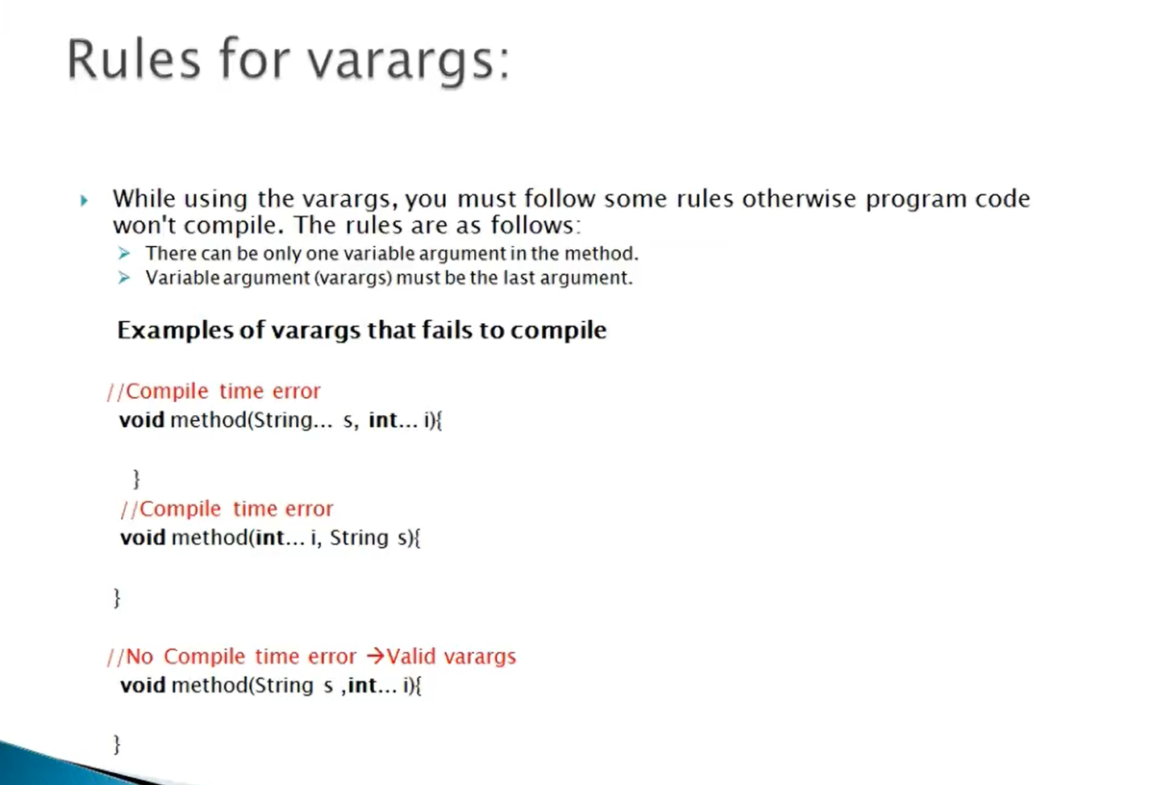
A crucial best practice when working with Java varargs is knowing when to use them and when to avoid them. Varargs excel when you need to handle a varying number of arguments, enhancing code flexibility and reducing repetition. However, they are not a universal solution. Consider varargs when argument counts genuinely fluctuate and simplify method calls. Conversely, in cases with fixed, known argument counts, traditional parameter lists may offer better efficiency and maintainability. Balancing varargs and fixed parameters ensures code that is both adaptable and high-performing.
Documenting Varargs Usage for Clarity
Clear and comprehensive documentation is a cornerstone of effective software development, and this holds true when working with varargs. Documenting varargs usage within your codebase is essential for enhancing code readability and aiding collaboration among developers. By providing clear comments and explanations, you ensure that the purpose and usage of varargs parameters are well-documented and understood by your team. This documentation helps developers quickly grasp how to use varargs in methods, reducing confusion and potential errors. Proper documentation of varargs empowers your team to work efficiently and maintain the codebase effectively, even as it evolves over time.
Monitoring and Measuring Performance Improvements
Optimizing code that involves varargs is an ongoing process, and it’s crucial to implement mechanisms for monitoring and measuring the effectiveness of your optimization efforts. Regularly monitoring your application’s performance using profiling tools and benchmarks allows you to assess the impact of optimizations accurately. By setting up performance metrics and measuring performance improvements, you can ensure that your code changes are delivering the desired results. This iterative approach not only helps in fine-tuning your code but also aids in detecting and addressing performance regressions that may arise as your codebase evolves. Monitoring and measurement are essential components of maintaining a high-performing application that incorporates varargs effectively.
Case Study: Refactoring for Performance
To provide a practical demonstration of how optimization techniques can be applied in the context of Java varargs, we present a detailed case study. In this case study, we take a sample code snippet that relies on varargs and systematically refactor it for improved performance. We’ll guide you through each step of the optimization process, highlighting the changes made and the rationale behind them. By following this case study, you’ll gain valuable insights into the real-world application of optimization techniques, allowing you to implement similar improvements in your own codebase. Furthermore, we will measure and showcase the tangible performance gains achieved through these refactoring efforts. This real-world example serves as a concrete illustration of how optimizing varargs can lead to more efficient and responsive Java applications, reinforcing the importance of balancing code flexibility with performance considerations.
Conclusion
Java varargs, while a powerful tool for code flexibility, come with performance considerations that must not be overlooked. By understanding the hidden costs of varargs and applying the optimization techniques and best practices outlined in this article, you can strike a balance between flexibility and efficiency in your Java code. Efficient code not only enhances performance but also contributes to a superior user experience. Take the initiative to apply these techniques in your Java projects, and your code will shine in terms of both flexibility and speed.