In the realm of Java programming, efficient data manipulation is crucial. When it comes to working with collections, the Map.containsKeySet.contains method combination offers a powerful tool for managing and retrieving data. In this comprehensive guide, we will delve into the intricacies of this method combo, exploring its functionality, use cases, and best practices for optimizing your code. By the end of this article, you’ll have a thorough understanding of how to harness the full potential of Map.containsKeySet.contains for improved performance and productivity.
Understanding Maps in Java
Before we dive into the specifics of Map.containsKeySet.contains, let’s establish a solid foundation by understanding what maps are in Java and why they are so essential for developers. Maps are data structures that store key-value pairs and provide efficient means to retrieve values based on their associated keys. Java offers various map implementations, such as HashMap, TreeMap, and LinkedHashMap, each with its own characteristics and use cases.
Map.containsKeySet.contains: The Basics
Map.containsKeySet.contains is a combination of three methods frequently used in Java programming to perform operations on maps. Let’s break down each part:
- Map: As mentioned earlier, a map is a collection that stores key-value pairs, where each key is unique;
- containsKey: This method checks whether a map contains a specific key;
- Set: A set is an unordered collection that contains unique elements;
- contains: This method checks whether a set contains a specific element.
Use Cases and Scenarios
Now that we understand the basic components let’s explore some common use cases and scenarios where Map.containsKeySet.contains can be particularly useful:
Data Validation
When it comes to user authentication, the combined power of containsKey, contains, and maps shines. Imagine a scenario where you need to validate user credentials securely. With containsKey, you can efficiently determine if a username exists in your user database, serving as the initial gatekeeper. Once you establish the user’s identity, you can then seamlessly employ contains to compare the provided password against the securely hashed and stored password, ensuring a robust authentication process.
Counting Occurrences
In the realm of text processing, containsKey and contains can elevate your word frequency tracking endeavors. Consider the task of analyzing a large document. By using containsKey, you can swiftly build a map to monitor word occurrences. As you process each word, you can efficiently employ contains to determine if a specific word has already made its appearance. This dynamic duo streamlines the process of counting word frequencies, making it invaluable for text analysis and natural language processing tasks.
Caching and Memoization
Efficiency is paramount when dealing with expensive function calls or complex calculations. Enter containsKey and contains to the rescue. Imagine a scenario where you need to perform intricate computations. By employing containsKey, you can quickly assess whether the result of a particular computation is already cached in a map. If it exists, contains enables you to retrieve the precomputed result instantly, sparing your system from redundant and time-consuming calculations. This caching and memoization strategy is a game-changer, optimizing performance and reducing computational overhead significantly.
Performance Considerations
While Map.containsKeySet.contains is a powerful tool, it’s essential to consider performance implications, especially for large datasets. Here are some performance considerations:
Time Complexity
Understanding the time complexity of these methods in different data structures is crucial for efficient programming:
- When you employ containsKey, you’re venturing into the world of time complexity. This method tends to perform exceptionally well with HashMaps, boasting a constant-time complexity of O(1). It’s lightning-fast, making it a top choice for tasks where quick key-based lookups are essential. However, in the realm of TreeMap, containsKey has a slightly different story to tell. Here, it exhibits a time complexity of O(log n), reflecting the log-linear nature of tree-based structures. This means that as the size of your TreeMap grows, the time taken for key lookups increases logarithmically;
- On the other hand, when you turn to contains, the story shifts. HashSet, a popular choice for its speed, excels with contains by offering constant-time complexity of O(1). This means that whether you’re checking for the existence of an element or verifying a set membership, it happens at breakneck speed. However, in the land of TreeSet, which maintains elements in sorted order, contains has a different tale to tell. Here, the time complexity spikes to O(n), linear in nature, as it has to traverse the entire set to confirm element presence. This makes TreeSet better suited for scenarios where sorted elements are a priority rather than lightning-fast lookups.
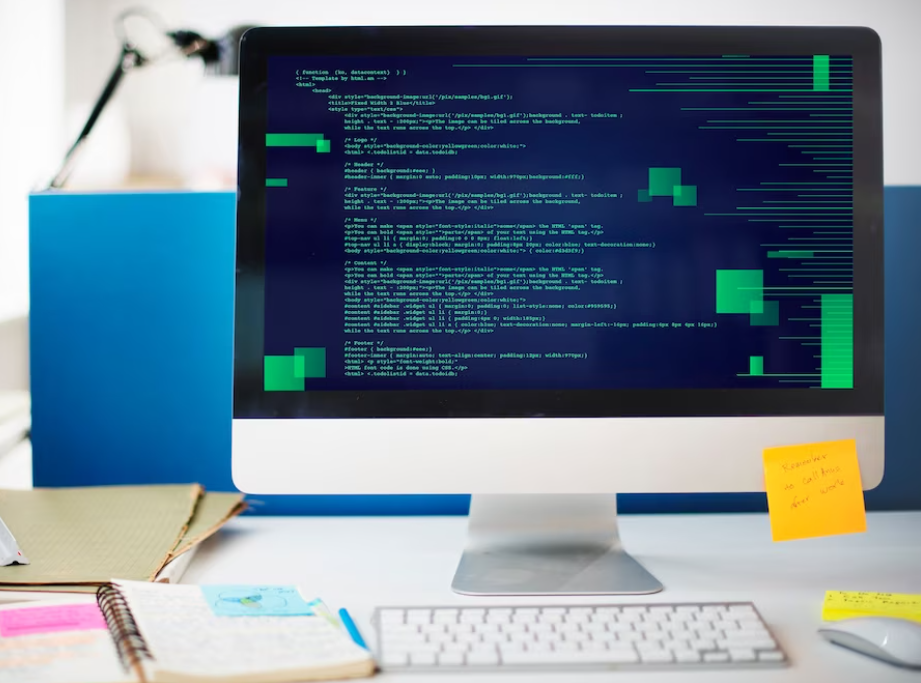
Memory Usage
Memory efficiency is a critical aspect to consider when choosing between these data structures:
- HashMaps, renowned for their efficiency in key-value storage, generally demand less memory than their counterpart, TreeMap. This is particularly valuable in situations where you aim to optimize memory consumption without sacrificing quick key-based access. HashMaps achieve this by using an array-based structure combined with hash codes, resulting in a balanced memory-performance trade-off;
- Likewise, when we venture into the world of sets, HashSet shines as the memory-efficient champion. Compared to TreeSet, HashSet consumes less memory. This is because HashSet doesn’t need to maintain the sorted order of elements, allowing it to save memory while still offering rapid set operations. This makes HashSet a preferred choice for scenarios where memory conservation is a priority, such as in applications with large datasets.
Best Practices and Code Examples
To make the most of Map.containsKeySet.contains, consider the following best practices:
Best Practice | Description |
---|---|
Choose the Right Map Implementation | Select the map type that suits your use case, balancing factors like retrieval speed and order maintenance. |
Optimize for Read-Heavy or Write-Heavy Operations | Tune your data structures for the predominant operation type in your application, such as reads or writes. |
Handle Concurrent Access | In multi-threaded environments, use ConcurrentHashMap to ensure thread safety and efficient scaling. |
Select Proper Data Structures | Beyond maps, choose the right set implementation (e.g., HashSet or TreeSet) based on your specific requirements. |
Profile and Benchmark | Continuously optimize your code by profiling for bottlenecks and benchmarking map implementations under different workloads. |
- First and foremost, choose the appropriate map implementation that suits your use case. containsKey operates differently across various map types like HashMap, TreeMap, and others, each with its own performance characteristics. Assessing your specific needs and selecting the right map type is crucial for optimal results;
- Ensure that your code accounts for potential null values when using containsKey. To prevent NullPointerExceptions, it’s good practice to check if a key is present in the map before attempting to retrieve its associated value;
- Keep performance in mind. If you anticipate frequent use of containsKey, consider optimizing your data structures and algorithms to minimize lookup times. This may involve selecting the most suitable map implementation or employing caching mechanisms.
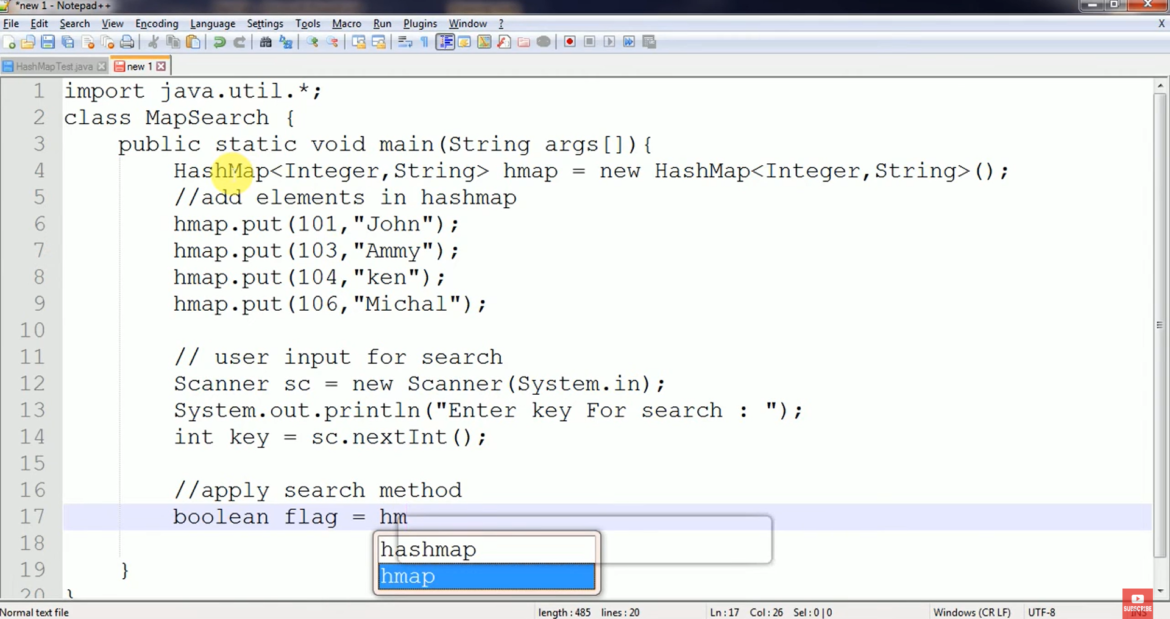
Example
To illustrate these best practices in action, let’s explore some code examples. In a user authentication scenario, you can utilize containsKey to validate user credentials by checking if a username exists in a user database. Here’s a simplified example:
Map<String, String> userDatabase = new HashMap<>();
userDatabase.put(“john_doe”, “hashed_password”);
String usernameToCheck = “john_doe”;
if (userDatabase.containsKey(usernameToCheck)) {
// Username exists, proceed with password validation
String hashedPassword = userDatabase.get(usernameToCheck);
// Perform password validation logic here
} else {
// Username does not exist, handle accordingly
System.out.println(“User not found.”);
}
In this code snippet, containsKey efficiently verifies the username’s existence in the userDatabase, demonstrating a practical application of this method in the context of user authentication.
Conclusion
Map.containsKeySet.contains is a valuable combination of methods that can significantly enhance your Java programming capabilities. By understanding its basics, exploring use cases, and considering performance considerations and best practices, you can leverage this combination to create efficient and robust applications. Remember that choosing the right map implementation, optimizing for your specific use case, and handling concurrency are key factors in making the most of this powerful tool. With the knowledge gained from this guide, you are well-equipped to navigate the world of Java collections with confidence and efficiency.