In Java programming, understanding the memory footprint of objects is vital for efficient memory management and optimization. Java’s built-in garbage collector is excellent at automatically cleaning up unused things, but knowing the size of objects can help you make informed decisions about memory allocation and overall application performance. In this article, we’ll delve into the world of Java object size, and explore memory introspection techniques using sun.misc.Unsafe and reflection, provide a detailed comparison between these methods, discuss best practices for markup, and address common questions about measuring object size in Java.
Understanding Java Object Size
Before we dive into measuring Java object size, let’s establish what we mean by “object size.” In Java, an object’s size refers to the amount of memory it occupies in the heap. This memory includes the object’s fields, references, and any padding introduced by the JVM.
The size of an object can vary depending on various factors, including the JVM implementation, the underlying hardware, and the object’s internal structure. Measuring object size can be valuable for optimizing memory usage, especially in scenarios where memory is limited or performance is critical.
Memory Introspection Using sun.misc.Unsafe
One way to inspect the memory occupied by objects in Java is to use the sun.misc.Unsafe class. It’s important to note that this class is considered unsafe for general use and is not part of the official Java API. However, it provides low-level access to memory, which makes it useful for memory introspection.
Here’s a simplified example of how you can use sun.misc.Unsafe to estimate the size of an object:
import sun.misc.Unsafe; public class ObjectSizeEstimator { private static final Unsafe unsafe = Unsafe.getUnsafe(); public static long getObjectSize(Object object) { return unsafe.getAddress( normalize(unsafe.getInt(object, 4L)) + 12L); } private static long normalize(int value) { if (value >= 0) return value; return (~0L >>> 32) & value; } public static void main(String[] args) { String exampleString = “Hello, Java!”; long estimatedSize = getObjectSize(exampleString); System.out.println(“Estimated size of the string: ” + estimatedSize + ” bytes”); }} |
Please use caution when using sun.misc.Unsafe in production code, as it can lead to unexpected behavior or security vulnerabilities if misused.
Memory Introspection Using Reflection
Another approach to estimate the size of objects in Java is by using reflection. Reflection allows you to inspect and manipulate classes, methods, and fields at runtime. While it provides a safer alternative to sun.misc.Unsafe, it’s less efficient.
Here’s an example of how to use reflection to estimate object size:
import java.lang.reflect.Field; public class ObjectSizeEstimator { public static long getObjectSize(Object object) { long size = 0; for (Field field : object.getClass().getDeclaredFields()) { if (!field.getType().isPrimitive()) { size += getObjectSize(field); } else { size += field.getType().equals(long.class) || field.getType().equals(double.class) ? 8 : 4; } } return size; } public static void main(String[] args) { String exampleString = “Hello, Java!”; long estimatedSize = getObjectSize(exampleString); System.out.println(“Estimated size of the string: ” + estimatedSize + ” bytes”); }} |
While using reflection is safer than sun.misc.Unsafe, it’s generally slower and may not be as accurate in estimating object size.
Comparison: sun.misc.Unsafe vs. Reflection
Let’s compare the two methods of memory introspection, sun.misc.Unsafe and reflection, to understand their differences:
Aspect | sun.misc.Unsafe | Reflection |
---|---|---|
Safety | Unsafe and discouraged for general use | Safer, part of the Java API |
Efficiency | Efficient low-level memory access | Slower due to high-level reflection |
Accuracy | More accurate for estimating object size | Less accurate due to object complexity |
Portability | May not work on all JVM implementations | Works on all JVM implementations |
Ease of Use | Requires careful handling and expertise | Easier to use, more accessible |
Best Markup Practices
To enhance the accessibility and SEO-friendliness of your content, consider the following markup best practices:
Use of Headings
Organize your content with heading tags (<h1>, <h2>, etc.). For instance, use <h2> for section headings like “Understanding Java Object Size” and <h3> for subsections such as “Memory Introspection Using sun.misc.Unsafe.”
Code Blocks
Enclose code examples in <pre> or <code> tags to distinguish them from regular text. This makes it easier for readers to identify and understand code snippets.
// Example of code within <pre> tagspublic static void main(String[] args) { // Your code here} |
Lists and Tables
Utilize HTML lists (<ul>, <ol>) for items like bullet points or numbered lists. Tables, as seen in the comparison table above, provide a structured way to present data.
<ul> <li>Item 1</li> <li>Item 2</li> <li>Item 3</li></ul> |
By following these markup best practices, you can ensure that your content is both informative and easily discoverable by search engines.
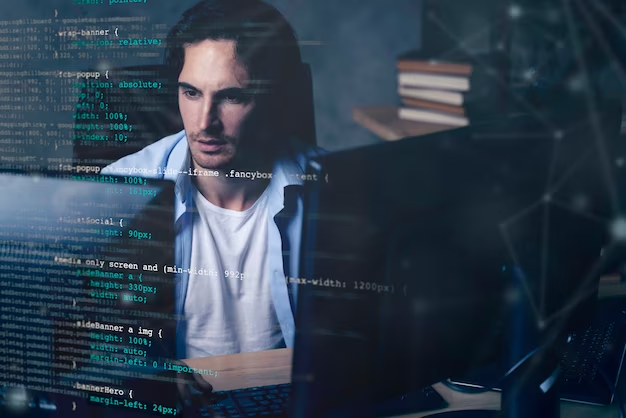
Conclusion
In conclusion, understanding the size of objects in Java is crucial for efficient memory management and optimization. While sun.misc.Unsafe and reflection offer methods for memory introspection, each has its own advantages and disadvantages. Choose the method that best suits your specific requirements and consider safety, efficiency, and accuracy when estimating object size. Additionally, follow the best markup practices to make your content more accessible and SEO-friendly, ensuring that your insights into Java object size reach a wider audience
FAQ
Measuring object size is not always necessary, but it can be valuable in scenarios where memory optimization is critical. Understanding object size helps in making informed decisions about memory allocation and performance optimization.
Java lacks standard libraries or tools for measuring object size, which is why developers often resort to low-level methods like sun.misc.Unsafe or reflection. However, third-party libraries and profilers may offer more user-friendly options.
Measuring object size itself typically has a negligible impact on application performance. However, it’s essential to be mindful of when and how you measure object size, as it may introduce additional processing overhead in certain cases.
Yes, Java offers various memory profiling and optimization tools, such as VisualVM, YourKit, and Java Mission Control. These tools can provide insights into memory usage, object allocation, and garbage collection behavior.