Memory leaks are a common concern in software development, and they can be especially tricky to spot and fix in complex data structures like multimap in C++. In this comprehensive guide, we will explore possible memory leaks in multimap and discuss effective strategies to detect and resolve them. By the end of this article, you’ll have a clear understanding of how to prevent memory leaks when working with multimaps in C++.
Understanding Multimaps
Multimaps in C++ are a versatile data structure that allows you to store and manage key-value pairs. They are similar to maps, but unlike maps, multimaps can contain multiple elements with the same key. This makes them useful for various applications, including maintaining sorted collections of data.
However, this flexibility comes with a trade-off. Mismanagement of memory in multimaps can lead to memory leaks, which occur when allocated memory is not properly deallocated, causing your program to consume more and more memory over time.
Causes of Memory Leaks in Multimaps
Memory leaks in multimaps typically occur due to one or more of the following reasons:
- Unreleased Memory: When you insert elements into a multimap, it allocates memory for each element. If you later erase elements or clear the multimap, you must ensure that the associated memory is properly deallocated. Failing to do so can result in memory leaks;
- Circular References: Multimaps can contain complex data structures as values. If these values have circular references or references to other dynamically allocated objects, it can create situations where memory is not released correctly, leading to leaks;
- Improper Iteration: Incorrect iteration through a multimap can result in memory leaks if you forget to release memory or delete elements properly during the iteration process;
- Exception Handling: Improper error handling or exception management can leave your multimap in an inconsistent state, preventing proper deallocation of memory.
Detecting Memory Leaks in Multimaps
Detecting memory leaks in multimaps can be challenging, but several strategies can help you identify and locate the leaks:
- Manual Inspection: Review your code carefully, paying attention to the allocation and deallocation of memory for multimap elements. Look for areas where memory may not be released properly;
- Memory Profiling Tools: Utilize memory profiling tools like Valgrind or AddressSanitizer. These tools can analyze your program’s memory usage and identify memory leaks, including those in multimaps;
- Custom Logging: Implement custom logging within your code to track memory allocation and deallocation for multimap elements. This can help you pinpoint the source of memory leaks;
- Automated Testing: Create automated test cases that stress test your multimap operations. Monitor memory usage during these tests to identify any abnormal memory consumption.
Resolving Memory Leaks in Multimaps
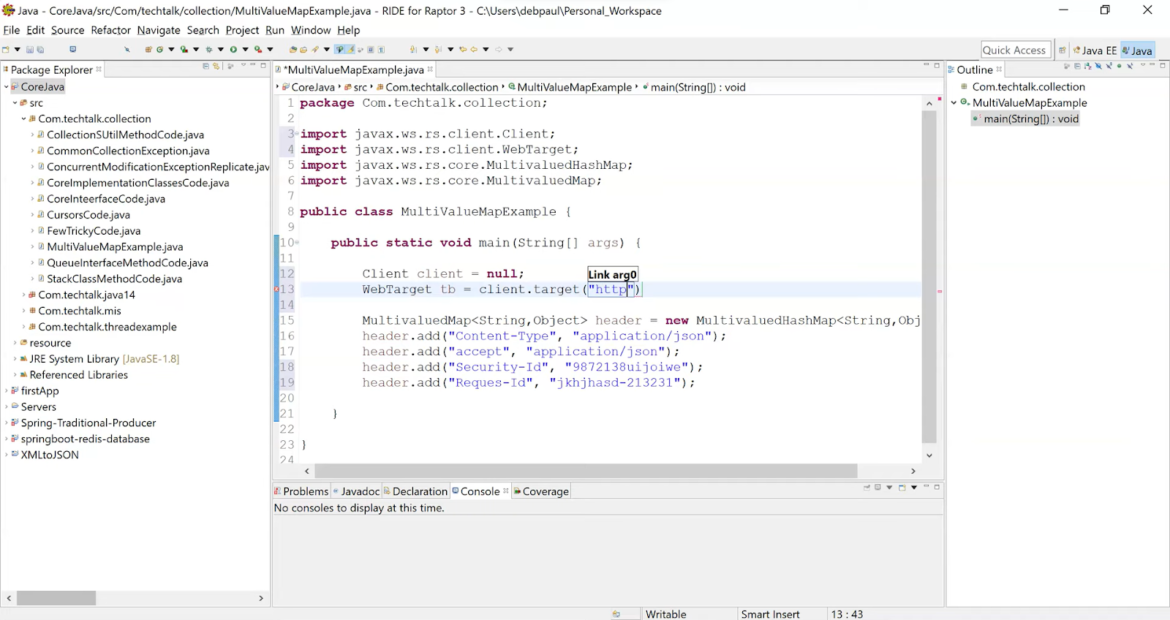
Once you’ve identified a memory leak in your multimap code, you can take steps to resolve it:
Proper Deallocation:
When you remove elements from your std::multimap, make sure to deallocate any dynamically allocated memory associated with those elements. If you’re storing pointers in the std::multimap, use delete or delete[] as appropriate to free the memory.
Smart Pointers:
Instead of managing memory manually with delete and delete[], consider using C++ smart pointers like std::shared_ptr or std::unique_ptr. These smart pointers automatically handle memory management, making it less error-prone and reducing the risk of memory leaks. For example, you can store std::shared_ptr or std::unique_ptr in your multimap, and the memory will be automatically released when it’s no longer needed.
Clearing and Rebuilding:
If you suspect that your multimap has circular references or complex ownership relationships that are causing memory leaks, you can clear the multimap and rebuild it when necessary. During this process, ensure that you properly deallocate memory as needed. Be cautious, though, as clearing and rebuilding might have performance implications, so use it judiciously.
Exception Safety:
Implement proper exception handling to ensure that memory is released correctly, even in error scenarios. If an exception is thrown during an operation involving your multimap, you should catch it and ensure that any allocated memory is properly deallocated in the exception handling code. This helps maintain the integrity of your program and prevents memory leaks.
Technique | Description |
---|---|
Proper Deallocation | Ensure correct memory deallocation for multimap elements when removed or when the multimap is destroyed. Use the delete or delete[] operator appropriately. |
Smart Pointers | Utilize C++ smart pointers (e.g., std::shared_ptr or std::unique_ptr) for automated memory management. -Smart pointers handle memory automatically, reducing memory leak risks. |
Clearing and Rebuilding | In cases of suspected circular references, clear the multimap and rebuild it to break any cycles. Ensure proper memory deallocation during the rebuilding process to maintain memory integrity. |
Exception Safety | Implement robust exception handling to ensure memory is released correctly, even during error scenarios. Prevent resources from being left in an inconsistent state. |
Best Practices to Prevent Memory Leaks
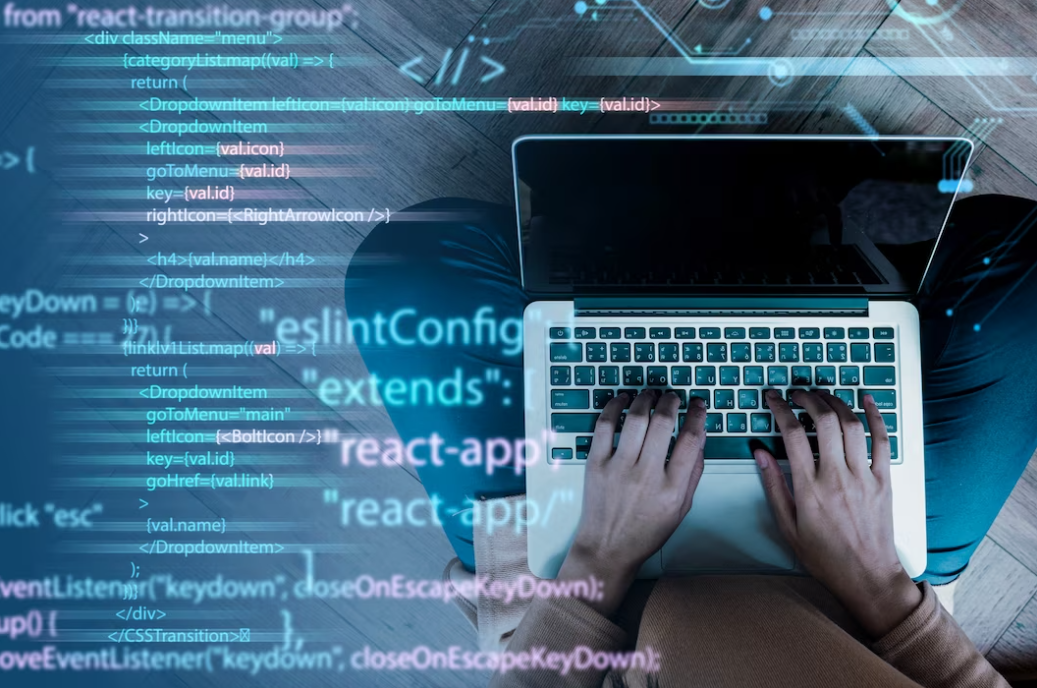
To prevent memory leaks from occurring in the first place, follow these best practices when working with multimaps:
- Use RAII Principles: Adhere to Resource Acquisition Is Initialization (RAII) principles, where resources, including memory, are managed by objects with well-defined lifetimes. This helps ensure that resources are automatically released when they go out of scope;
- Test Thoroughly: Perform thorough testing, including boundary tests and edge cases, to validate the correctness of your multimap operations and memory management;
- Code Review: Engage in code reviews with your team to catch potential memory leaks early in the development process;
- Utilize Modern C++ Features: Leverage modern C++ features like move semantics and smart pointers to simplify memory management and reduce the chances of leaks.
Conclusion
Memory leaks in multimaps can be a challenging issue to tackle, but with the right strategies and best practices, you can minimize the risk and effectively detect and resolve them. Remember to pay close attention to memory allocation and deallocation, use smart pointers where appropriate, and thoroughly test your code to ensure it remains leak-free. By following these guidelines, you can confidently work with multimaps in C++ without worrying about memory leaks affecting your applications.