In the realm of Java programming, a common obstacle that arises is the requirement to concatenate integers and strings. This article aims to provide a complete exploration of the different methodologies and strategies employed in Java for the purpose of concatenating integers with strings. The intricacies, best practices, and appropriate contexts for utilizing different approaches in the context of “adding int to string Java” will be explored, facilitating the development of a comprehensive comprehension.
Understanding the Basics
To effectively add integers to strings in Java, it’s crucial to grasp the fundamental concepts. Two primary techniques will be covered extensively in this article: using the + operator and leveraging the String.valueOf() method. Let’s start by understanding the core principles.
The + Operator
The + operator is the most straightforward way to combine integers and strings in Java. When you use + between a string and an integer, Java automatically converts the integer to its string representation and concatenates it with the existing string.
String.valueOf()
Another method involves explicitly converting the integer to a string using the String.valueOf() method. This method accepts an integer as input and returns its string representation.
Method | Description |
---|---|
String.valueOf(int i) | Returns the string representation of the integer i. |
Both techniques yield the same result: the seamless merging of integers and strings in Java.
Using the + Operator
Let’s delve deeper into the practical implementation of using the + operator to add integers to strings in Java.
Concatenating User Input
Consider a scenario where you’re building a program that asks the user for their age and then displays a message using that information.
import java.util.Scanner; public class AgeMessage { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print(“Please enter your age: “); int age = scanner.nextInt(); String message = “You are ” + age + ” years old.”; System.out.println(message); scanner.close(); } } |
In this example, the + operator is used to add the user’s age (an integer) to the string “You are” and ” years old.” The resulting message is displayed on the console.
Combining Variables
Suppose you have two variables, firstName and lastName, containing the first and last names of a person, and you want to create a full name string.
String firstName = “John”; String lastName = “Doe”; String fullName = firstName + ” ” + lastName; System.out.println(“Full Name: ” + fullName); |
Here, the + operator is employed to concatenate the first name, a space character, and the last name to form the fullName string.
Using + with Arithmetic
In some situations, you might need to incorporate arithmetic operations while adding integers to strings.
int x = 5; int y = 7; String result = “The sum of ” + x + ” and ” + y + ” is ” + (x + y); System.out.println(result); |
This example demonstrates how to add the result of an arithmetic operation to a string using the + operator. Note the use of parentheses to ensure the proper order of operations.
Leveraging String.valueOf()
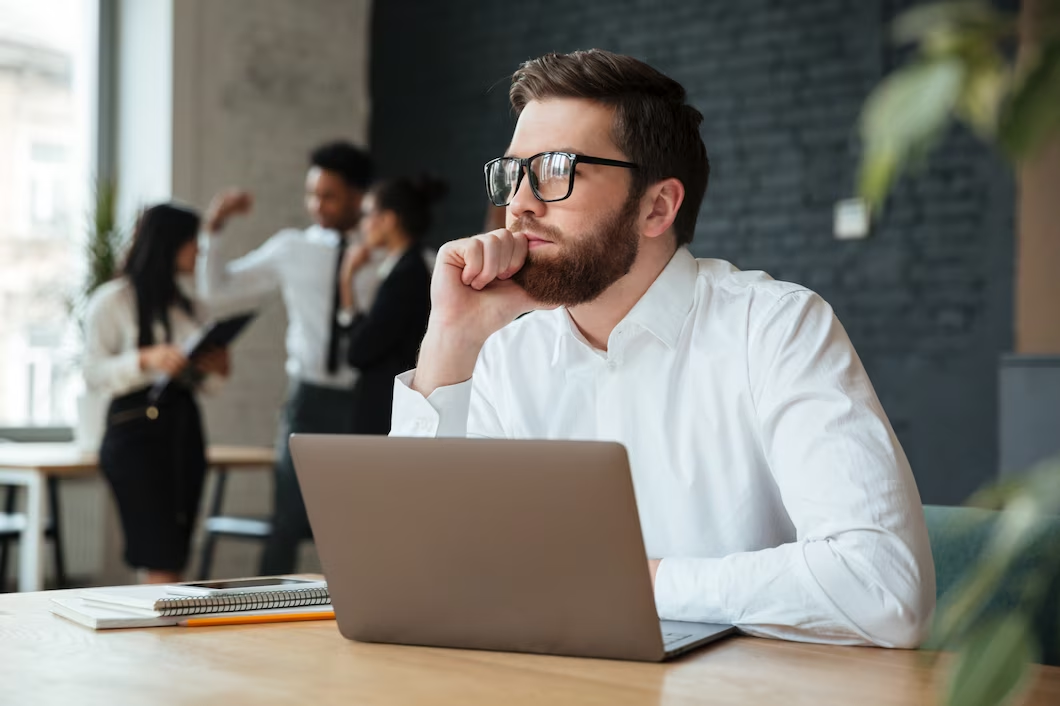
Now, let’s explore how to add integers to strings by leveraging the String.valueOf() method.
In Java, you can add integers to strings by leveraging the String.valueOf() method. This method is commonly used to convert primitive data types, including integers, into their string representations. Let’s explore how to use String.valueOf() to add integers to strings effectively.
Explicit Conversion
Suppose you have an integer num, and you want to create a string representation of it. You can achieve this using the following code snippet:
int num = 42; String numStr = “The number is: ” + String.valueOf(num); System.out.println(numStr); |
In this example, String.valueOf(num) explicitly converts the integer num to its string representation. The + operator is then used to concatenate this string with the rest of the message. The output of this code will be:
The number is: 42 |
Efficiency Matters: StringBuilder
While the + operator and String.valueOf() are suitable for most scenarios, they may not be the most efficient options when dealing with extensive string manipulations. That’s where StringBuilder comes into play.
StringBuilder is a mutable class that provides better performance for building large strings, especially when concatenating within loops or complex operations. Let’s examine its usage.
Concatenating Within a Loop
Suppose you need to concatenate a series of integers into a single string within a loop.
int[] numbers = {1, 2, 3, 4, 5}; StringBuilder builder = new StringBuilder(); for (int num : numbers) { builder.append(num).append(“, “); } String result = “Numbers: ” + builder.toString(); System.out.println(result); |
In this example, the StringBuilder object builder efficiently accumulates the integer values, and the final string is constructed using builder.toString().
Handling Null Values
In real-world scenarios, you might encounter situations where the integer value could be null. Handling such cases is essential to prevent unexpected behavior in your program.
Suppose you have an integer nullableNum that could be null, and you want to create a string representation of it.
Integer nullableNum = null; String numStr = “The number is: ” + (nullableNum != null ? String.valueOf(nullableNum) : “N/A”); System.out.println(numStr); |
In this example, a conditional statement is used to check if nullableNum is null. If it is, “N/A” is used as the string representation; otherwise, the integer is converted to a string.
Localization and Internationalization
When working on applications that require support for multiple languages or regions, it’s crucial to consider localization and internationalization. Java provides tools like MessageFormat and String.format() to facilitate string concatenation while accommodating various locales.
Suppose you want to display a message that includes an integer value in a format suitable for different languages.
import java.text.MessageFormat; import java.util.Locale; int quantity = 5; String itemName = “apple”; Locale currentLocale = Locale.getDefault(); MessageFormat messageFormat = new MessageFormat(“You have {0, number} {1}.”); String localizedMessage = messageFormat.format(new Object[] { quantity, itemName }, currentLocale); System.out.println(localizedMessage); |
In this example, MessageFormat is used to create a localized message by specifying placeholders for the integer and string values. This approach ensures that your application can adapt to different languages and cultural conventions.
Conclusion
In the vast landscape of Java programming, “adding int to string Java” is a fundamental operation that you’ll encounter in countless scenarios. This article has meticulously covered the techniques, best practices, and considerations involved in seamlessly merging integers and strings in Java.
Whether you choose the simplicity of the + operator, the explicitness of String.valueOf(), or the efficiency of StringBuilder, you are now well-equipped to handle this common task with confidence and expertise. Furthermore, we’ve explored how to handle null values, optimize performance with StringBuilder, and address localization needs for international applications.
By mastering these techniques, you’ll elevate your Java programming skills and be prepared to tackle a wide range of real-world challenges. So, go forth and craft dynamic, informative messages, and applications with ease.
FAQs
Yes, you can add other data types to strings using similar approaches. For instance, you can concatenate floating-point numbers, characters, or even custom objects by converting them to their string representations.
Yes, there can be a performance difference, particularly when dealing with extensive string manipulations. StringBuilder is generally more efficient for complex concatenations because it avoids creating unnecessary intermediate string objects.
Attempting to concatenate null with a string will result in the string “null” being added to the original string. To avoid this, ensure that the value you’re concatenating is not null or explicitly handle null values in your code.
Yes, you can add multiple integers to a string in a single statement by using the + operator multiple times. For example:
int a = 1;
int b = 2;
int c = 3;
String result = “The sum of ” + a + “, ” + b + “, and ” + c + ” is ” + (a + b + c);
Just ensure that you use parentheses when performing arithmetic operations to get the correct result.