Regular expressions, commonly shortened to regex or regexp, wield considerable power within the realm of text processing and manipulation. They empower users to establish precise patterns for locating and scrutinizing text within strings, furnishing a flexible mechanism for extracting, altering, or validating data. While the regex patterns themselves hold significance, multiple string methods, incorporated into various programming languages, harness the potential of regex to augment string manipulation capabilities. In the forthcoming article, we will delve into a selection of paramount regex-related string manipulation methods, unveiling their capacity to streamline intricate tasks.
Regular Expressions
Before we plunge into the intricacies of string methods harnessing regular expressions, let’s take a moment to revisit the fundamentals of regular expressions. At its core, a regular expression is a sequence of characters that serves as a blueprint for searching. It possesses the capability to locate, explore, and manipulate strings in alignment with precise criteria. Within the realm of regular expressions, you encounter an array of elements, comprising literal characters, metacharacters, and quantifiers. These components harmoniously intermingle to construct intricate patterns, expanding the horizons of text processing possibilities.
The .match() Method
One of the most fundamental string methods that utilizes regular expressions is the .match() method. This method allows you to search a string for a specified pattern and returns an array of all matched substrings. Here’s a simple example in JavaScript:
const text = "The quick brown fox jumps over the lazy dog";
const pattern = /quick|fox/g;
const matches = text.match(pattern);
console.log(matches);
// Output: ["quick", "fox"]
In this example, the regular expression /quick|fox/g searches for either “quick” or “fox” in the input text. The .match() method returns an array containing all matching substrings.
The .replace() Method
The .replace() method is another indispensable tool that makes use of regular expressions. It allows you to replace matched substrings with a specified replacement string. Consider the following example in Python:
import re
text = "Hello, world! This is a test."
pattern = r"\b\w{4}\b"
replacement = "****"
new_text = re.sub(pattern, replacement, text)
print(new_text)
# Output: "Hello, ****! This is a ****."
In this case, the regular expression \b\w{4}\b matches four-letter words, and the .replace() method substitutes them with asterisks.
The .split() Method
The .split() method is incredibly handy when you need to split a string into an array of substrings based on a specified delimiter. Regular expressions can be used as delimiters for more complex splitting scenarios. Here’s an example in Python:
import re
text = "apple,banana;cherry.orange"
pattern = r"[,;.]"
result = re.split(pattern, text)
print(result)
# Output: ['apple', 'banana', 'cherry', 'orange']
In this example, the regular expression [,;.] is used to split the input text wherever a comma, semicolon, or period is encountered.
The .search() Method
The .search() method is used to search for a specified pattern within a string and returns the position of the first occurrence. If no match is found, it returns -1. Here’s an example in Java:
import java.util.regex.*;
public class Main {
public static void main(String[] args) {
String text = "The quick brown fox jumps over the lazy dog";
String pattern = "quick|fox";
Pattern compiledPattern = Pattern.compile(pattern);
Matcher matcher = compiledPattern.matcher(text);
if (matcher.find()) {
System.out.println("Match found at position: " + matcher.start());
// Output: Match found at position: 4
} else {
System.out.println("Match not found");
}
}
}
In this Java example, the regular expression quick|fox is used to search for either “quick” or “fox” in the input text, and the .search() method returns the position of the first match.
The .matchAll() Method
The .matchAll() method, available in some programming languages like JavaScript, takes regex to the next level. It returns an iterator that yields all matches in the string, including capture groups. This method is incredibly powerful when you need to extract structured data from a text. Here’s an example in JavaScript:
const text = "John Doe (30 years old) and Jane Smith (25 years old)";
const pattern = /(\w+\s\w+)\s\((\d+) years old\)/g;
for (const match of text.matchAll(pattern)) {
console.log(`Name: ${match[1]}, Age: ${match[2]}`);
}
In this example, the regular expression captures names and ages from the input text using capture groups, and .matchAll() iterates through all the matches, allowing you to process them individually.
The .test() Method
The .test() method is a simple yet powerful tool for regex-based validation. It checks if a string contains a match for a specified pattern and returns a boolean value. Here’s an example in Python:
import re
pattern = r"^\d{3}-\d{2}-\d{4}$"
valid_ssn = "123-45-6789"
invalid_ssn = "1234-567-890"
print(re.search(pattern, valid_ssn).group()) # Output: "123-45-6789"
print(re.search(pattern, invalid_ssn)) # Output: None
# Using .test() method for validation
print(re.search(pattern, valid_ssn) is not None) # Output: True
print(re.search(pattern, invalid_ssn) is not None) # Output: False
In this Python example, the regular expression ^\d{3}-\d{2}-\d{4}$ validates Social Security Numbers (SSNs), and the .test() method checks if the SSN is valid.
Performance Considerations
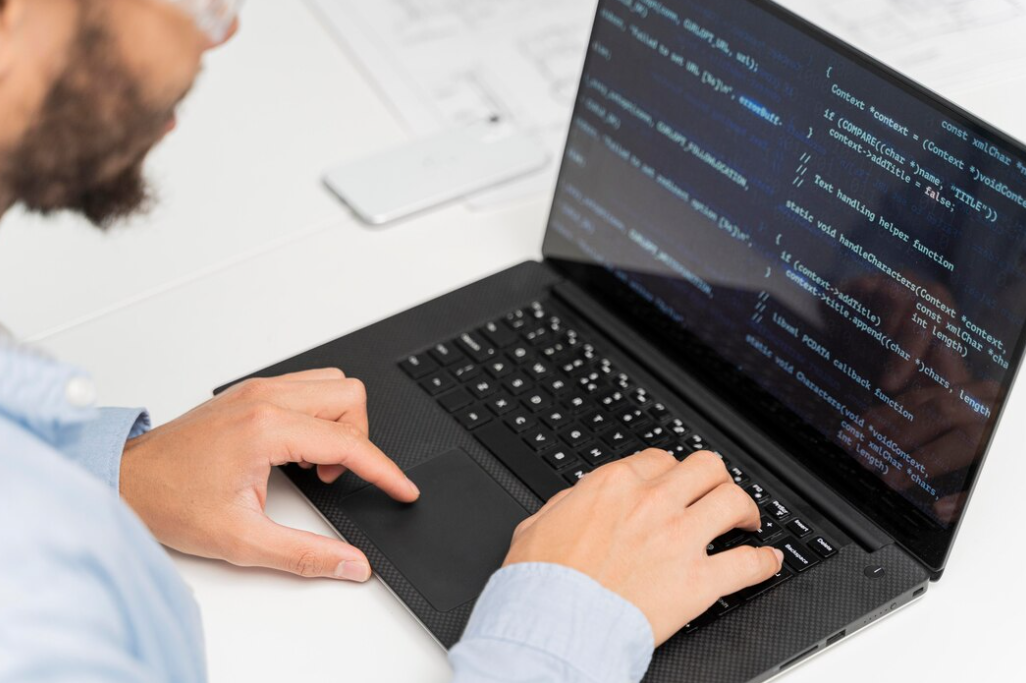
When optimizing Java Regex performance, several key considerations can significantly impact the efficiency and responsiveness of your applications. Here are some essential points to keep in mind:
- Regex Pattern Complexity: Complex regex patterns, especially those with nested quantifiers or extensive backtracking, can lead to substantial performance overhead. Be cautious when crafting intricate patterns, and consider simplifying them when possible;
- Code Profiling: Utilize profiling tools to identify performance bottlenecks related to regex usage in your code. Profiling helps pinpoint which parts of your application consume the most processing time, allowing you to focus your optimization efforts effectively;
- Pattern Optimization: Optimize your regex patterns by simplifying them and using more efficient constructs. Non-capturing groups, possessive quantifiers, and atomic groups can reduce unnecessary backtracking, improving overall regex efficiency;
- Regex Object Caching: Cache regex objects, especially in scenarios where patterns are reused. Creating and compiling regex patterns can be resource-intensive, so caching precompiled patterns can save processing time and resources;
- Input Data Size: Consider the size of the input data you are processing. Regex operations on large texts can be more time-consuming than on smaller ones. Implement strategies to handle large datasets efficiently;
- Testing and Benchmarking: Regularly test and benchmark your regex-based code to measure its performance under different scenarios. Benchmarking helps you identify areas for improvement and track the impact of optimization efforts;
- Avoid Overfitting: Avoid overly complex regex patterns that may be “overfitted” to your specific data. A more generalized regex may perform better across various inputs.
Conclusion
Regular expressions are a potent tool for string manipulation, and the regexp-related methods of string handling in various programming languages open up a world of possibilities. Whether you need to search, replace, split, validate, or extract data from strings, regular expressions provide a flexible and efficient solution. However, it’s essential to use them judiciously and optimize your patterns for performance when working with large datasets. By mastering these regexp-related string methods, you’ll become a more effective text-processing programmer, capable of tackling a wide range of challenges in data manipulation and validation.