The grasp of constructors assumes a paramount significance in the realm of Java programming. Constructors, being a specialized breed of method, wield the exceptional power to instigate objects, thereby endowing them with their foundational attributes. Within the intricate tapestry of Java, one encounters a particular variety known as the “Java static constructor.” Astonishingly, this variant often resides in the shadows, overlooked by many Java artisans, despite its latent utility in precise contexts. This treatise endeavors to embark on a profound exploration of the enigmatic realm of Java static constructors. It shall delve into its precise delineation, apt applications, and distinctive attributes, juxtaposed against their traditional counterparts.
What is a Java Static Constructor?
In the Java programming language, a constructor stands as a distinct method with the singular purpose of initializing objects that belong to a particular class. These constructors come into play when an instance of a class is brought to life through the invocation of the “new” keyword. The fundamental aim of this ritual is to ensure that the newly created object commences its existence in a state that aligns with predefined standards, rendering it poised for immediate use.
In stark contrast, the Java static constructor possesses its own unique characteristics. Often referred to as a “static initialization block,” this construct diverges significantly from conventional constructors. Unlike their counterparts, static constructors are not tethered to specific instances of a class but are, instead, tethered to the class as a whole. They serve as a conduit for executing class-level initialization procedures, typically a one-time affair.
Here’s how you can define a static constructor in Java:
public class MyClass { static { // Static constructor code here } } |
The code inside the static block will be executed once, and only once, when the class is loaded by the Java Virtual Machine (JVM). This makes static constructors a powerful tool for performing class-level initialization tasks.
Key Differences Between Regular and Static Constructors
Java static constructors play a unique role in the initialization of classes compared to regular constructors. To comprehend their significance, it is crucial to distinguish between the two. Here, we outline the primary distinctions:
1. Association with Instances vs. Classes
- Regular Constructors: These constructors are tightly linked to instances of a class. They get invoked whenever a new object is created using the new keyword. Essentially, they cater to instance-level initialization;
- Static Constructors: In contrast, static constructors are associated with the class itself, not individual instances. They execute when the class is loaded by the Java Virtual Machine (JVM), making them suitable for class-level initialization tasks.
Let’s delve deeper into each of these aspects:
2. Execution Trigger
- Regular Constructors: Every time a new object is created, regular constructors are triggered. They initialize instance-specific data for each object separately;
- Static Constructors: Static constructors, on the other hand, are executed only once during class loading. This ensures that class-level initialization is performed just once, regardless of how many instances of the class are created.
3. Accessibility
- Regular Constructors: For regular constructors, you have the flexibility to specify access modifiers such as public, private, protected, or package-private (default access). This allows you to control the visibility and accessibility of the constructor;
- Static Constructors: Similar to regular methods, you can also specify access modifiers for static constructors. This allows you to manage their accessibility within the class and from external sources.
4. Parameters
- Regular Constructors: Regular constructors can accept parameters, which are often used to initialize instance variables. These parameters are passed when creating a new object, making it possible to customize the object’s initial state;
- Static Constructors: Static constructors, in their conventional form, do not directly accept parameters. Instead, they are primarily used to initialize static fields or perform other class-level tasks that don’t rely on instance-specific data.
5. Initialization Context
- Regular Constructors: Regular constructors have access to the specific instance being created. This grants them the ability to initialize instance variables based on the characteristics of that particular object. They can tailor the object’s properties to its unique requirements;
- Static Constructors: In contrast, static constructors do not have access to any specific instance. They are associated with the class as a whole. Consequently, they are suitable for tasks that do not depend on instance-specific data. Static constructors are often employed for tasks like initializing static fields or setting up class-wide configurations.
When to Use Java Static Constructors
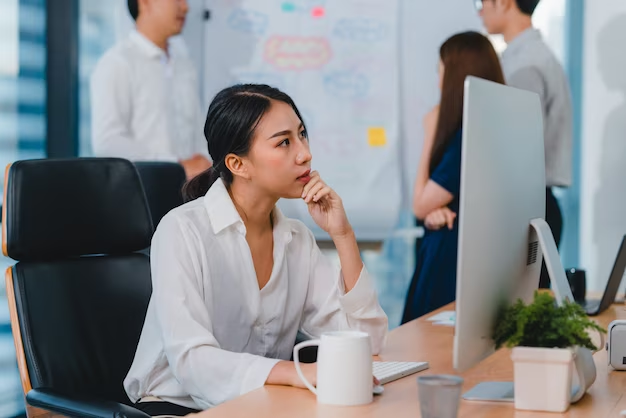
Now that you understand the differences between regular and static constructors, let’s explore when it’s appropriate to use static constructors in your Java code:
1. Initializing Static Fields
One common use case for static constructors is initializing static fields within a class. Static fields belong to the class, not to instances, and they are shared among all instances of the class. If you have complex initialization logic for static fields, a static constructor is an ideal place to put it.
public class Configuration { public static String baseUrl; static { // Initialize the static baseUrl field baseUrl = loadBaseUrlFromConfigFile(); } } |
2. Loading Native Libraries
If your Java application relies on native libraries, you can use static constructors to load these libraries when the class is loaded. This ensures that the native libraries are available when needed.
public class NativeLibraryLoader { static { System.loadLibrary(“myNativeLibrary”); } } |
3. Initializing Class-Level Resources
In some cases, you might need to initialize resources or perform other class-level tasks when a class is loaded. Static constructors provide a clean way to do this.
public class ResourceManager { private static List<Resource> resources; static { // Initialize the resources list resources = loadResourcesFromDatabase(); } } |
4. Configuration and Setup
Static constructors are also useful for configuring and setting up your application or framework. You can use them to read configuration files, initialize logging, or perform other global setup tasks.
public class MyApp { static { // Initialize application-wide settings configureLogging(); } } |
5. Singleton Pattern
In some cases, you may want to implement the Singleton design pattern using a static constructor to ensure that only one instance of a class is created.
public class Singleton { private static Singleton instance; private Singleton() { // Private constructor to prevent direct instantiation } static { // Initialize the Singleton instance instance = new Singleton(); } public static Singleton getInstance() { return instance; } } |
Conclusion
In the world of Java programming, constructors play a vital role in object initialization. While regular constructors are well-known and widely used, static constructors often remain underutilized despite their significant advantages. They provide a means to perform class-level initialization tasks, making them a powerful tool in your Java development toolbox.
By understanding the differences between regular and static constructors and knowing when to use each, you can enhance the structure and efficiency of your Java code. Whether you’re initializing static fields, loading native libraries, or configuring your application, Java static constructors are there to help you streamline the process and ensure your classes are ready for action. So, don’t overlook this valuable feature in your Java programming journey.
FAQs
Yes, a class can have both a static constructor (static initialization block) and one or more regular constructors. The static constructor is responsible for class-level initialization, while regular constructors are used to initialize instances of the class.
The static constructor is executed when the class is loaded by the Java Virtual Machine (JVM). It runs once and only once, ensuring that class-level initialization tasks are performed.
No, you cannot pass parameters directly to a static constructor. Static constructors do not have access to instance-specific data. However, you can use static methods with parameters to achieve similar functionality.
Static constructors are not inherited by subclasses. Each class with a static constructor is responsible for its own class-level initialization. Subclasses can have their own static constructors if needed.
If an exception is thrown inside a static constructor, the class will not be successfully loaded by the JVM. This can lead to NoClassDefFoundError or other class-loading-related errors.
No, it’s not always necessary to define a static constructor. If you don’t provide one, the JVM will still perform a default class-level initialization, which typically involves setting static fields to their default values (e.g., null for objects, zero for primitives).
Static constructors are executed by the JVM in a thread-safe manner, ensuring that they are run only once, even in a multithreaded environment. However, it’s essential to be cautious when performing class-level initialization tasks that involve shared resources.