Java is renowned for its flexibility in handling various data types and objects. One of the essential skills every Java programmer should possess is the ability to convert strings into other objects effortlessly. In this comprehensive guide, we’ll walk you through the intricacies of string packing and demonstrate how to convert strings to any other objects in Java.
Introduction to String Packing
String packing, also known as converting strings to objects, is a fundamental skill in Java programming. It allows developers to transform textual data into various object types, enabling them to work with the information efficiently. Let’s delve into the basics before exploring more complex conversions.
The Basics of Java Strings
Before we dive into conversions, it’s crucial to understand Java strings. Strings are sequences of characters enclosed in double quotes, such as “Hello, World!”. In Java, strings are objects of the String class, providing numerous methods for manipulation.
Converting Strings to Integers
Using Integer.parseInt()
The most common conversion is from strings to integers. Java provides the Integer.parseInt() method for this purpose. Here’s an example:

Handling Number Format Exceptions
Be cautious when converting; if the string doesn’t represent a valid integer, a NumberFormatException is thrown. You should always handle such exceptions gracefully.
String to Double Conversion
Converting strings to double values follows a similar pattern. You can use Double.parseDouble():

Parsing Strings to Dates
Parsing strings into date objects is a frequent task in applications dealing with timestamps. Java’s SimpleDateFormat class simplifies this process:

Converting Strings to Custom Objects
Sometimes, you may need to convert strings into custom objects. This requires implementing your own conversion logic based on the string format and the target object’s structure.

Handling Exception Cases
As mentioned earlier, handling exceptions is crucial when converting strings to objects. Robust error handling ensures your application doesn’t crash when unexpected data is encountered.
String Packing Best Practices
To excel in string packing, adhere to these best practices:
- Validate input strings;
- Use try-catch blocks to handle exceptions;
- Document your conversion methods thoroughly;
- Unit test your conversion code extensively.
Advanced Techniques
For more advanced scenarios, consider exploring:
- Regular expressions for complex string patterns;
- Custom type converters using libraries like Jackson or Gson.
Common Pitfalls to Avoid
When working with string packing in Java, it’s essential to be aware of common pitfalls that can lead to errors or inefficient code. Here are some pitfalls to avoid:
Insufficient Data Validation
Failing to validate input strings adequately can result in unexpected behavior or exceptions. Always validate and sanitize user-provided data before attempting conversions.
Neglecting Locale Considerations
When converting strings to numeric or date values, consider the user’s locale. Different locales may use different number formats or date representations.
Not Handling Time Zones
When dealing with date and time conversions, ensure you account for time zones correctly. Neglecting time zone considerations can lead to inaccurate results.
Poorly Optimized Code
Inefficient conversion code can impact the performance of your application. Optimize your conversion logic to minimize resource usage and execution time.
String Packing in Java vs. Other Languages
While string packing is a common task in many programming languages, the approach can vary. Here’s a comparison table illustrating how Java compares to some other popular languages when it comes to converting strings to objects:
Language | String to Integer Conversion | String to Date Conversion | Custom Object Conversion |
---|---|---|---|
Java | Integer.parseInt() | SimpleDateFormat | Custom conversion code |
Python | int() | strptime() | Custom conversion code |
JavaScript | parseInt() | new Date() | Custom conversion code |
C# | int.Parse() | DateTime.ParseExact() | Custom conversion code |
Tips for Optimizing String Packing Performance
To ensure your Java applications run efficiently when handling string packing, consider implementing these performance optimization tips:
Use StringBuilder for String Concatenation
When building strings from multiple parts, use StringBuilder instead of string concatenation with the + operator. StringBuilder is more efficient as it avoids unnecessary string copying.
Precompile Regular Expressions
If you use regular expressions for string parsing, precompile them using Pattern.compile() to improve performance, especially when parsing multiple strings.
Employ Multithreading for Parallel Processing
In scenarios where you need to convert a large number of strings simultaneously, consider using multithreading to parallelize the conversion tasks, making the process faster.
Optimize Data Structures
Choose appropriate data structures for storing intermediate or final results. For example, use a HashMap for quick lookups when mapping strings to custom objects.
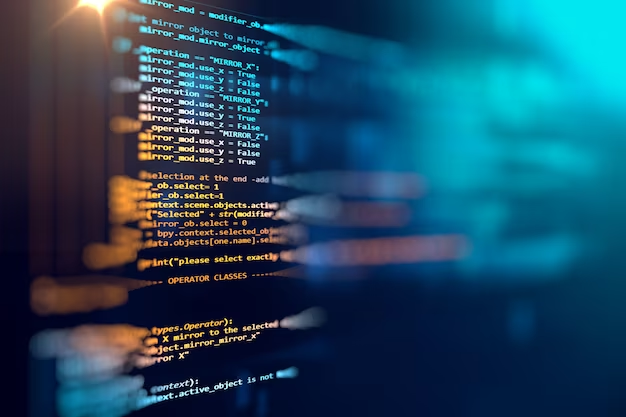
Resources for Further Learning
To deepen your understanding of string packing and Java programming, here are some valuable resources:
- Oracle’s Java Documentation: Explore the official Java documentation to learn more about the String class and its methods;
- Online Java Courses: Enroll in online courses or tutorials to enhance your Java skills, focusing on string manipulation and conversions;
- Java Forums and Communities: Join Java programming forums and communities to seek advice, share knowledge, and stay updated on best practices.
Handling Null and Empty Strings
In real-world scenarios, you’ll often encounter null or empty strings when dealing with user input or data from external sources. Handling these situations gracefully is crucial to prevent unexpected behavior. Here’s how to manage null and empty strings in your string packing endeavors:
- Null Strings: When you receive a null string, check for it explicitly before attempting any conversions. Trying to parse or convert a null string will result in a NullPointerException. You can use a simple if statement to safeguard your code.

- Empty Strings: Empty strings (those with zero length) may also require special handling depending on your use case. For instance, you may want to treat them as zero when converting to numeric types or provide a default value.
Treating Empty Strings as Default Values

- Logging and Error Handling: When handling null or empty strings, it’s a good practice to log warnings or errors to help diagnose potential issues in your application. Proper logging can save you time troubleshooting problems later.
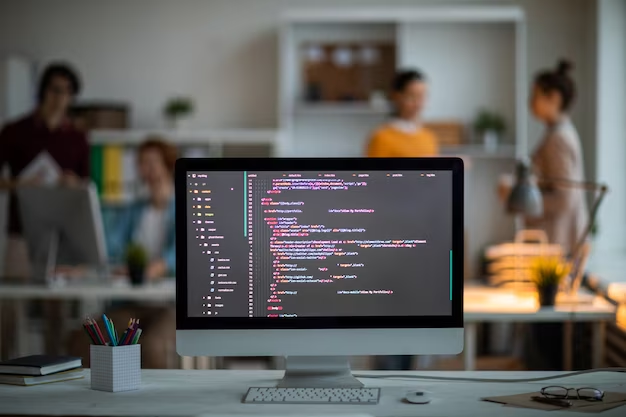
String Packing Use Cases
String packing, although seemingly straightforward, has numerous practical applications in Java programming. Here are some common use cases where you might find string packing invaluable:
Data Validation
String packing is often used in data validation routines. For instance, verifying that a user-provided input matches an expected format, such as validating email addresses or phone numbers.
File Parsing
When reading data from files, you may need to convert strings representing file contents into appropriate data types. This is particularly common when parsing CSV, JSON, or XML files.
User Interfaces
In graphical user interfaces, user inputs are typically in string form. String packing is necessary to convert these inputs into the appropriate data types for processing.
Database Interaction
When interacting with databases, data retrieved often arrives as strings. String packing is vital for transforming this data into usable objects within your Java application.
Web APIs and Serialization
When working with web APIs or serialization/deserialization of data, converting strings into objects is a critical step in exchanging information between systems.
Popular Libraries for String Manipulation in Java
Library | Description | Pros | Cons |
---|---|---|---|
java.lang.String | Standard Java library for string manipulation. | Widely available, no additional setup | Limited functionality |
StringBuilder | Efficient for building strings incrementally. | Excellent performance for concatenation | Limited methods for manipulation |
StringUtils (Apache Commons) | Provides various string utility methods. | Extensive functionality, battle-tested | Requires external library dependency |
java.util.regex | Java’s built-in regular expression library. | Powerful pattern matching | Steeper learning curve |
Conclusion
Mastering string packing, the art of converting strings to any other objects in Java, is a valuable skill for any Java developer. By understanding the fundamentals, handling exceptions, and following best practices, you can harness the power of data manipulation in your Java applications.
FAQs
When converting strings to objects, common exceptions include NumberFormatException for invalid numeric conversions and ParseException for date conversions.
Yes, you can convert strings into custom objects by parsing and mapping the string data to the object’s attributes.
Advanced techniques include using regular expressions for complex string patterns and employing custom type converters with libraries like Jackson or Gson.
Error handling ensures that your application gracefully handles unexpected data, preventing crashes and enhancing overall robustness.
You can validate input strings by checking their format, length, and other criteria using conditional statements and regular expressions.