In the realm of Java programming, the implementation of exception handling stands as an eminent imperative. It empowers developers to adeptly address unanticipated errors, ensuring the steadfastness and dependability of their software endeavors. Within the domain of Java programming, a pivotal role is shouldered by the “throw” statement in orchestrating this intricate maneuver. The intention of this composition is to embark on a comprehensive exploration of the process associated with the hurling of exceptions within the Java programming lexicon. This discourse shall delve into the intricacies of syntax, endorsed methodologies, and the customary impediments that accompany this facet of Java programming. By the time you conclude this exhaustive odyssey, you shall have acquired the requisite competencies to dexterously employ the “throw exception java” capability within your Java-based undertakings.
Understanding Exceptions in Java
Prior to exploring the throw statement, it is imperative to get a comprehensive understanding of exceptions in the Java programming language. Exceptions refer to unforeseen occurrences or mistakes that arise while a program is being executed, so disturbing its typical progression. Errors can arise due to a range of circumstances, including but not limited to, invalid input, file unavailability, or division by zero. In order to address these exceptional cases, Java has a strong system that incorporates the use of the toss statement.
What is an Exception?
An exception in Java is a runtime error or an event that disrupts the normal flow of a program. Exceptions can occur for a multitude of reasons, including:
- Trying to access an array index that doesn’t exist;
- Opening a file that does not exist;
- Dividing a number by zero;
- Attempting to access an object that is null;
- Invalid input data.
The throw Statement
The utilization of the “throw” statement stands as a deliberate action to cast forth an exception. This particular construct empowers developers with the means to signify the occurrence of a distinctly exceptional scenario nestled within their codebase. When the “throw” statement is wielded, it necessitates the provision of an instance derived from an exception class, thereby serving as a vessel for the intricate details pertaining to the exception’s category and the contextual backdrop in which it unfurls.
Syntax of the throw Statement
The syntax for using the throw statement in Java is straightforward:
throw throwableObject; |
Here, throwableObject is an instance of a class that extends the Throwable class, which is the base class for all exceptions in Java. You can throw any exception type that is a subclass of Throwable.
Example of Using the throw Statement
Let’s illustrate the usage of the throw statement with a simple example. Suppose you are building a calculator program, and you want to prevent division by zero. You can throw an ArithmeticException when a division by zero is attempted:
public class Calculator { public double divide(int numerator, int denominator) { if (denominator == 0) { throw new ArithmeticException(“Division by zero is not allowed.”); } return (double) numerator / denominator; } } |
In this example, if the denominator is zero, the throw statement is executed, creating an ArithmeticException and providing a custom error message.
Checked vs. Unchecked Exceptions
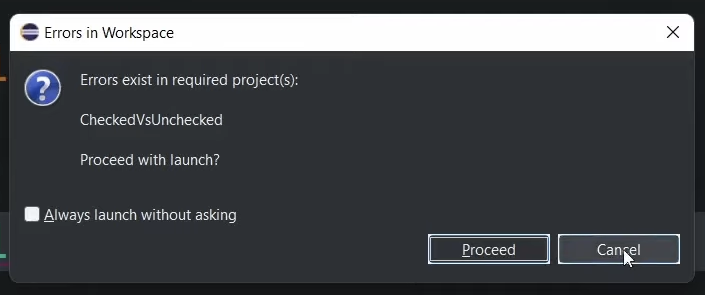
Exceptions within the Java programming lexicon assume a pivotal role in orchestrating the response to unforeseen circumstances or glitches that may transpire during the course of program execution. They can be neatly compartmentalized into two fundamental classifications: the realm of checked exceptions and the domain of unchecked exceptions, each fulfilling a unique function within Java’s intricate exception handling apparatus.
Checked Exceptions
In the Java programming language, checked exceptions refer to exceptions that necessitate either being caught or declared by the invoking method through the use of the throws keyword. The purpose of these exceptions is to symbolize external circumstances that necessitate your code to handle them in a composed manner, frequently involving interactions with external resources or services. When a method raises a checked exception, it is indicating to the caller that it must be equipped to handle potential exceptional circumstances.
Common examples of checked exceptions include:
- IOException: This exception occurs when there is an issue with input or output operations, such as reading from or writing to files or network connections. It is often necessary to catch or declare this exception when dealing with file operations;
- SQLException: When working with databases, SQLException can be thrown to indicate issues like connection problems or incorrect SQL queries. It’s essential to handle this exception properly when dealing with database interactions.
Checked exceptions play a vital role in ensuring robust error handling, especially when your code interacts with external resources or services that might fail unpredictably. Handling these exceptions appropriately helps prevent unexpected program crashes and ensures a graceful response to exceptional situations.
Unchecked Exceptions
Unchecked exceptions, alternatively referred to as runtime exceptions, are a category of exceptions that do not necessitate explicit declaration through the throws keyword, nor are they obligatory to be caught. These exceptions generally signify programming flaws or problems that can be mitigated through code examination and appropriate validation. Unchecked exceptions are frequently linked to defects or logical problems in the code.
Examples of unchecked exceptions include:
- NullPointerException: This exception occurs when your code attempts to access an object or invoke a method on an object that is null. It’s a common mistake that can be avoided through careful programming and null checks;
- ArrayIndexOutOfBoundsException: This exception arises when you try to access an array element using an index that is outside the valid range. Proper array bounds checking can prevent this issue.
Unchecked exceptions are not subject to explicit checking by the compiler, hence potentially resulting in unforeseen software behavior if not appropriately handled. Although it is not mandatory, it is considered a best practice to capture and manage unchecked exceptions in order to improve error messaging and boost the overall resilience of your system.
Best Practices for Using throw
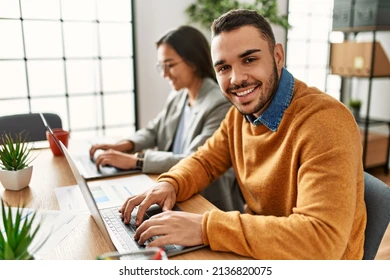
To make the most of the throw statement in Java, it’s essential to follow best practices to ensure clean and maintainable code.
- Use the Appropriate Exception Type: Choose the most specific exception type that accurately describes the exceptional condition. Using the correct exception type makes it easier to handle exceptions gracefully and understand the nature of the problem;
- Provide Descriptive Error Messages: When throwing an exception, include a clear and informative error message. This message should help developers understand the cause of the exception and how to resolve it. Descriptive error messages make debugging and troubleshooting more manageable;
- Document Exception Handling: In your code, document the exceptions that a method can throw using the @throws Javadoc tag. This practice helps other developers understand the expected exceptions and how to handle them;
- Handle Exceptions Appropriately: Always handle exceptions in your code, either by catching them and taking appropriate action or by declaring them using the throws keyword in the method signature. Avoid ignoring exceptions, as this can lead to unexpected program behavior;
- Use Try-Catch Blocks: Use try-catch blocks to catch and handle exceptions. This allows you to gracefully recover from exceptional conditions and maintain the normal flow of your program. Here’s an example:
try { // Code that may throw an exception } catch (SomeException e) { // Handle the exception } |
Common Pitfalls
When working with exceptions and the throw statement in Java, there are some common pitfalls that developers should be aware of:
Common Exception Handling Mistakes | Description |
---|---|
Throwing Generic Exceptions | Avoid throwing generic exceptions like Exception or RuntimeException without a specific reason. These catch-all exceptions can make debugging difficult and lead to unexpected behavior in your code. |
Overusing Checked Exceptions | While checked exceptions are essential for handling exceptional conditions, overusing them can lead to verbose and cluttered code. Only use checked exceptions when there is a clear need for them. |
Catching and Swallowing Exceptions | Catching exceptions without taking any action, also known as swallowing exceptions, can hide underlying issues and make it challenging to diagnose problems in your code. Always handle exceptions appropriately or let them propagate up the call stack. |
Not Logging Exceptions | Failing to log exceptions can make troubleshooting and debugging difficult, especially in production environments. Use logging frameworks like Log4j or SLF4J to record exceptions and their context. |
Conclusion
The management of exceptional conditions is a crucial component of Java programming that contributes to its robustness. The throw statement, in particular, serves as a potent tool in this regard. By adhering to established guidelines and circumventing typical errors, one may guarantee that Java programs effectively manage exceptions, hence enhancing user satisfaction and facilitating the process of identifying and resolving issues. It is advisable to incorporate the throw exception statement in Java programming, employing it judiciously to enhance the dependability and manageability of one’s code.
FAQs
throw is used to explicitly throw an exception within a method.
throws is used in a method declaration to indicate that the method may throw one or more specific exceptions, which should be handled by the caller.
Yes, you can create your custom exception classes by extending the Exception class or one of its subclasses, such as RuntimeException. This allows you to define and throw exceptions tailored to your application’s specific needs.
You can catch multiple exceptions in a single catch block by using the vertical bar (|) to separate the exception types. For example:
try {
// Code that may throw exceptions
} catch (IOException | SQLException e) {
// Handle IOException or SQLException
}
No, you are not required to catch or declare all exceptions in Java. Checked exceptions must be caught or declared, but unchecked exceptions (runtime exceptions) do not need to be explicitly caught or declared.
You should rethrow an exception in Java when you want to propagate it up the call stack without handling it at the current level. This allows higher-level code to deal with the exception appropriately.