When it comes to data manipulation and storage in Java, serialization plays a crucial role. Serialization allows you to convert Java objects into a binary format for easy storage, transmission, and retrieval. In this comprehensive guide, we will explore various methods of binary serialization in Java, from the built-in Java Object Serialization to third-party libraries and custom implementations. Whether you’re a novice Java developer or an experienced coder looking to deepen your knowledge, this article will provide valuable insights into the world of binary serialization.
Understanding Binary Serialization
Binary serialization involves converting Java objects into a binary format, allowing you to store or transmit them efficiently. This process is crucial when you need to persist data or send it across a network. Understanding the different methods available for binary serialization in Java will empower you to make informed decisions based on your project’s requirements.
Java Object Serialization
Java Object Serialization is the built-in mechanism for serializing Java objects. It allows you to save and restore the state of an object. Here’s a simple example of how to use it:
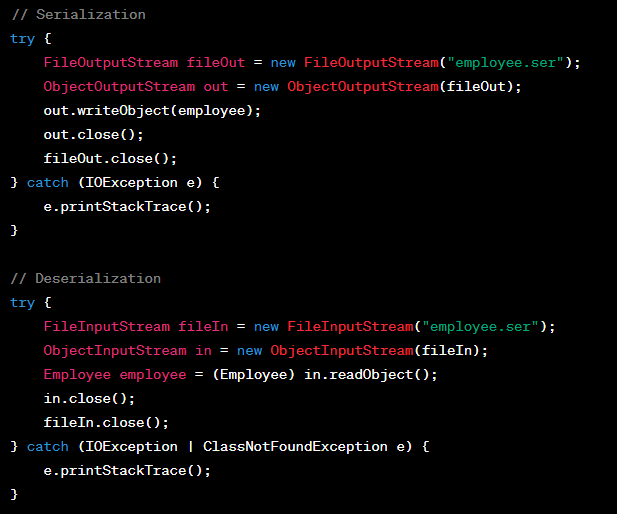
Externalizable Interface
For more control over serialization, you can implement the Externalizable interface. This interface allows you to customize the serialization and deserialization process by providing writeExternal and readExternal methods. It’s suitable for complex objects that require special handling.
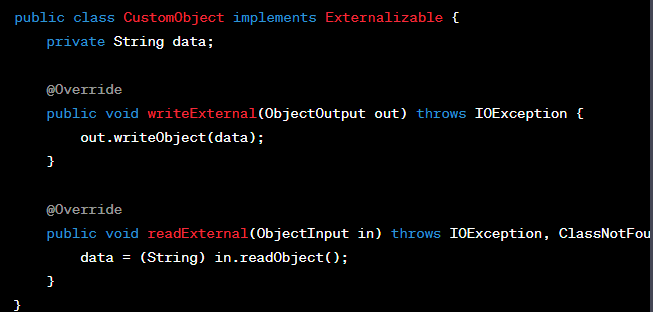
Jackson Library for Binary Serialization
When working with JSON data, the Jackson library is a powerful tool for binary serialization. It can convert Java objects to JSON and vice versa with ease. Here’s a quick example of serialization with Jackson:
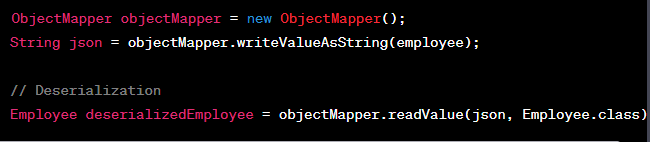
Protocol Buffers (ProtoBuf)
Protocol Buffers, or ProtoBuf, provide a highly efficient binary serialization format. Google developed this technology for use in various applications. It offers a compact binary representation and is ideal for inter-process communication.
To use ProtoBuf in Java, you need to define a .proto file and use the ProtoBuf compiler to generate Java code for serialization and deserialization.
Custom Binary Serialization
In some cases, you may need to implement custom binary serialization to meet specific requirements. This involves manually encoding and decoding objects into binary data. While it offers maximum flexibility, it can be more complex and time-consuming than using built-in serialization methods.
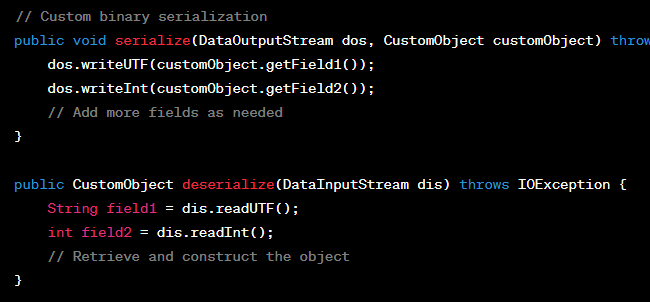
Performance Comparison
The choice of serialization method can significantly impact the performance of your Java application. Factors like speed, size of serialized data, and ease of use should be considered. Here’s a performance comparison of the discussed methods:
Method | Speed | Data Size | Ease of Use |
---|---|---|---|
Java Object Serialization | Moderate | Larger | Easy |
Externalizable Interface | Customizable | Custom | Moderate |
Jackson Library | Fast | Compact | Easy |
Protocol Buffers (ProtoBuf) | Very Fast | Compact | Moderate |
Custom Serialization | Varies | Varies | Custom |
The choice depends on your specific project requirements and trade-offs between speed, data size, and complexity.
Tips and Best Practices
When working with binary serialization in Java, keep the following tips and best practices in mind:
- Versioning: Be cautious about backward and forward compatibility of serialized objects;
- Security: Consider security aspects, especially when deserializing data from untrusted sources;
- Compression: Use data compression if you need to reduce the size of serialized data;
- Testing: Always test serialization and deserialization thoroughly to ensure correctness.
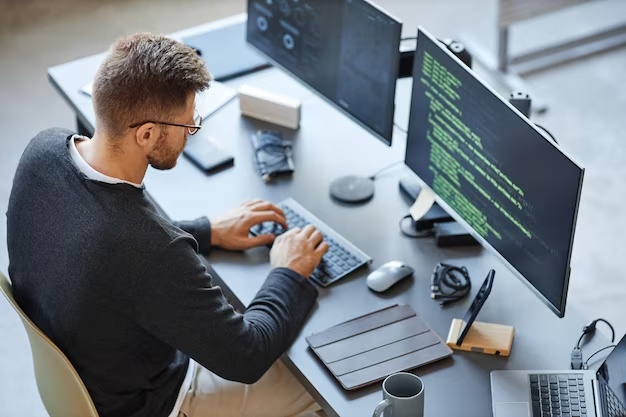
Serialization and Security
Serialization in Java can introduce security risks, particularly when deserializing data from untrusted sources. Here are some security considerations:
Common Security Issues in Serialization
- Deserialization Vulnerabilities: Attackers may exploit vulnerabilities in the deserialization process to execute malicious code;
- Data Tampering: Serialized data can be intercepted and tampered with during transmission if not properly secured;
- Versioning and Compatibility: Changes in the object’s structure between versions can lead to unexpected behavior and security issues.
Best Practices for Secure Serialization
To enhance security during serialization:
Practice | Description |
---|---|
Use Input Validation | Validate incoming serialized data to prevent malicious input. |
Implement Custom Serialization | Control the serialization process to limit security risks. |
Encrypt Serialized Data | Encrypt serialized data to protect it during transmission. |
Keep Libraries Updated | Update serialization libraries to patch known vulnerabilities. |
Serialization Performance Tips
Serialization can impact the performance of your Java application. Here are some tips to optimize performance:
Tips for Improving Serialization Performance
- Choose the Right Method: Select the serialization method that best balances speed and data size for your use case.
- Avoid Unnecessary Serialization: Serialize only the data needed, excluding transient and unnecessary fields.
- Use Compression: Apply data compression techniques to reduce the size of serialized data.
- Optimize Object Graphs: Minimize the depth and complexity of object graphs to speed up serialization.
Serialization Performance Comparison
Let’s revisit the performance comparison table and focus on performance-related metrics:
Method | Speed | Data Size | Ease of Use | Performance Tips |
---|---|---|---|---|
Java Object Serialization | Moderate | Larger | Easy | Optimize object graphs, avoid unnecessary fields |
Externalizable Interface | Customizable | Custom | Moderate | Implement custom serialization |
Jackson Library | Fast | Compact | Easy | Minimize object depth, use compression |
Protocol Buffers (ProtoBuf) | Very Fast | Compact | Moderate | Optimize object graphs, use compression, choose ProtoBuf for speed |
Serialization in Distributed Systems
In distributed systems, binary serialization is essential for transmitting data efficiently between nodes. Here’s how it plays a vital role:
Benefits of Binary Serialization in Distributed Systems
- Reduced Bandwidth: Binary serialization produces compact data, reducing the amount of data transmitted over the network;
- Faster Transmission: Smaller data payloads lead to quicker data transmission, improving overall system performance;
- Interoperability: Binary serialization allows different platforms and languages to exchange data seamlessly.
Future Trends in Binary Serialization
The world of binary serialization in Java is continually evolving. Here are some future trends to watch for:
Emerging Trends in Binary Serialization
- Cloud-Native Serialization: Serialization techniques tailored for cloud-native applications to enhance scalability and flexibility;
- Blockchain Integration: Serialization methods adapted for blockchain-based data storage and smart contracts;
- Quantum-Safe Serialization: Preparing for future quantum computing threats by ensuring the security of serialized data.
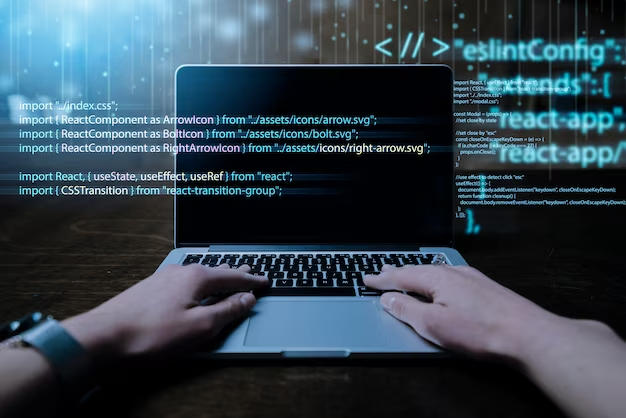
Conclusion
In this article, we’ve delved into the various methods of binary serialization in Java, ranging from the built-in Java Object Serialization to custom solutions and third-party libraries like Jackson and ProtoBuf. Each method has its strengths and weaknesses, making it essential to choose the one that best fits your project’s requirements. By understanding these serialization techniques, you can optimize data storage, transmission, and retrieval in your Java applications.
FAQs
Binary serialization in Java is the process of converting Java objects into a binary format for efficient storage, transmission, and retrieval.
Custom binary serialization is useful when you need fine-grained control over the serialization process or have specific requirements not met by built-in methods.
Java Object Serialization is built into Java and is easy to use but may produce larger data. ProtoBuf offers a highly efficient binary format but requires defining a schema.
Versioning your serialized objects and handling changes gracefully is essential for compatibility.
The speed of serialization methods varies, with ProtoBuf typically being the fastest, followed by Jackson, depending on the specific use case.