In the world of software development, efficient data storage and retrieval are paramount. Large-scale applications often require handling massive amounts of data, making data structures like hashmaps crucial for optimal performance. In this post, we will dive deep into five popular hashmap implementations: the Java Development Kit (JDK), Fastutil, Goldman Sachs HPPC, Koloboke, and Trove. By the end of this article, you’ll have a clear understanding of their features, advantages, and when to use each one in your projects.
Java Development Kit (JDK) Hashmap
The Java Development Kit (JDK) includes a powerful data structure known as a HashMap, which is a fundamental part of Java’s Collections Framework. A HashMap is essentially a key-value store that allows efficient storage and retrieval of data. It is known for its excellent performance characteristics and is widely used in various applications.
One of the key advantages of the JDK’s built-in HashMap is its fast retrieval time. It uses a hashing mechanism to store and locate objects based on their keys, making lookups almost constant time on average, regardless of the size of the map. This makes it ideal for scenarios where you need quick access to data based on unique keys.
HashMaps find their use in a multitude of scenarios, including caching, data indexing, and data retrieval. They are particularly valuable in scenarios where you need to associate values with unique keys and want to ensure quick access to these values without iterating through a collection.
Here’s an example of how to use a HashMap in Java:
import java.util.HashMap;
public class HashMapExample {
public static void main(String[] args) {
// Creating a HashMap
HashMap<String, Integer> studentScores = new HashMap<>();
// Adding key-value pairs
studentScores.put("Alice", 95);
studentScores.put("Bob", 88);
studentScores.put("Charlie", 92);
// Retrieving values
int aliceScore = studentScores.get("Alice");
System.out.println("Alice's score: " + aliceScore);
// Checking if a key exists
boolean hasKey = studentScores.containsKey("Dave");
System.out.println("Does Dave have a score? " + hasKey);
// Iterating through the HashMap
for (String name : studentScores.keySet()) {
int score = studentScores.get(name);
System.out.println(name + ": " + score);
}
}
}
Best practices when using HashMap:
- Ensuring that keys are immutable and that objects used as keys override the hashCode() and equals() methods properly;
- Be aware that HashMaps are not thread-safe by default, so if you plan to use them in a multi-threaded environment, consider using ConcurrentHashMap or synchronizing access to the HashMap to prevent concurrent modification issues;
- Monitor the HashMap’s load factor and consider resizing it if it becomes too full to maintain optimal performance.
Fastutil Hashmap
Fastutil, a renowned Java library, excels in enhancing performance and memory efficiency through specialized collection classes. Notably, it offers a high-performance hashmap implementation that surpasses the standard JDK HashMap.
Fastutil’s hashmap prioritizes memory conservation and performance optimization. Achieving this by specializing in primitive data types like int, long, and char, it excels in efficiently storing and handling such data. This specialization minimizes memory overhead, a notable improvement over the JDK HashMap, which deals with objects.
One of Fastutil’s significant advantages lies in its speed. Thanks to specialization and optimized algorithms, it consistently outperforms the standard HashMap, particularly when managing primitive data types. This makes it an excellent choice for high-performance data storage and retrieval tasks.
In benchmarks and real-world usage, Fastutil’s hashmap excels, especially in numerical computation, data processing, and scientific computing. When managing substantial datasets of primitive data, it notably reduces memory usage and provides faster access times than the JDK HashMap.
Here’s an example of how to use Fastutil’s hashmap for storing integers:
public class FastutilHashMapExample {
public static void main(String[] args) {
// Creating a Fastutil hashmap for integers
Int2IntOpenHashMap studentScores = new Int2IntOpenHashMap();
// Adding key-value pairs
studentScores.put(1, 95);
studentScores.put(2, 88);
studentScores.put(3, 92);
// Retrieving values
int aliceScore = studentScores.get(1);
System.out.println("Alice's score: " + aliceScore);
// Checking if a key exists
boolean hasKey = studentScores.containsKey(4);
System.out.println("Does Dave have a score? " + hasKey);
// Iterating through the Fastutil hashmap
studentScores.forEach((key, value) -> System.out.println("Student " + key + ": " + value));
}
}
Goldman Sachs HPPC (High-Performance Primitive Collections) Hashmap
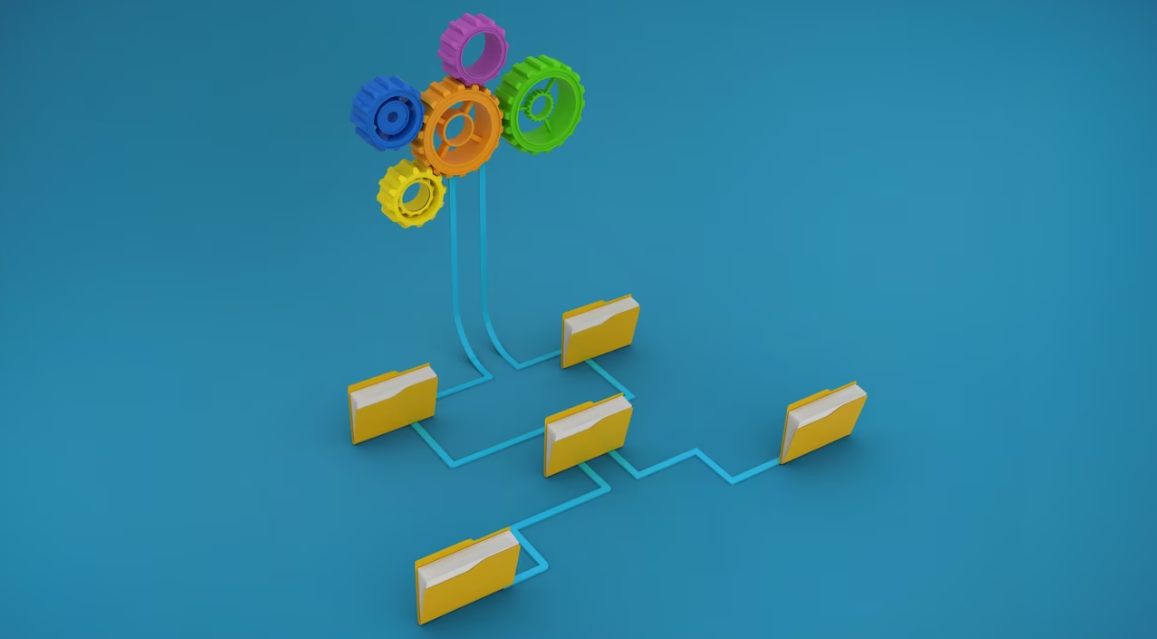
Goldman Sachs’ High-Performance Primitive Collections (HPPC) Hashmap is a remarkable addition to the world of data structures and collections. Taking an in-depth look at HPPC’s hashmap capabilities reveals its exceptional design and efficiency. Unlike traditional hashmap libraries, HPPC is meticulously crafted to handle primitive data types with unparalleled speed and memory efficiency. It is optimized to deliver exceptional performance for scenarios where data size and processing speed are critical.
- HPPC excels in high-throughput, low-latency applications, making it ideal for finance, where speed is paramount. It outperforms competitors in handling numerical data, enabling financial institutions to process vast datasets swiftly;
- HPPC’s design prioritizes memory efficiency, a crucial factor in large-scale data operations. By specializing in primitive types, it minimizes overhead from wrapper classes like Integer or Double, resulting in more efficient memory usage.
Feature/ Aspect | HPPC Hashmap | Java’s HashMap | Guava’s MapMaker | Trove’s THashMap |
---|---|---|---|---|
Performance | High-speed, optimized for primitives | Good for general-purpose use | Moderate, not specialized | Specialized for primitives |
Memory Efficiency | Excellent, minimal object overhead | Moderate, increased object overhead | Moderate, increased object overhead | Good, minimal object overhead |
Primitive Support | Exceptional, specialized for primitive types | Requires autoboxing/unboxing | Requires autoboxing/unboxing | Specialized for primitive types |
Concurrency Support | Limited, not designed for concurrent use | Requires external synchronization | Limited, not designed for concurrent use | Limited, not designed for concurrent use |
Ease of Use | May require more code for primitive handling | Easy to use for general data types | Easy to use for general data types | May require more code for primitive handling |
Customization | Limited customization options | Limited customization options | Moderate customization options | Moderate customization options |
Community Support | Smaller community, primarily used in finance | Large and established Java community | Moderate-sized community | Smaller community, specialized |
Use Cases | High-throughput financial applications | General-purpose Java applications | General-purpose Java applications | Specialized scenarios with primitive data |
License | Apache License 2.0 | Oracle Binary Code License | Apache License 2.0 | Open-source licenses |
Koloboke Hashmap
Koloboke, a popular Java library, offers a range of highly efficient hashmap options, making it a go-to choice for developers seeking optimized data structures. Here’s a closer look at what Koloboke has to offer:
Koloboke provides a variety of hashmap implementations tailored for different use cases, including hash tables, open-addressing, and separate chaining. It is known for its emphasis on memory efficiency and performance optimization, making it a valuable addition to any Java project requiring efficient data storage.
- Memory Efficiency and Speed: Koloboke excels in both memory efficiency and speed. Its hashmap implementations are designed to minimize memory overhead, making it an excellent choice for applications dealing with large datasets. Moreover, Koloboke’s hashmaps are crafted for high performance, with optimizations aimed at reducing cache misses and ensuring swift data access;
- Use Cases: Koloboke is well-suited for a wide range of applications. Its memory-efficient design makes it particularly valuable in resource-constrained environments. Developers working on projects involving data caching, in-memory databases, or any application where memory and speed are crucial can benefit from Koloboke’s offerings.
Trove Hashmap
Trove’s hashmap solutions provide a comprehensive and specialized approach to data management in Java. These offerings stand out for their unwavering focus on memory efficiency and performance optimization. By specializing in primitive data types, Trove significantly reduces memory overhead, making it an invaluable asset in resource-constrained environments. The elimination of autoboxing and unboxing results in rapid data access, ideal for high-throughput applications where minimal latency is essential. Trove’s hashmaps have found real-world utility in diverse fields, from finance to gaming, and are praised for their ability to handle extensive numerical or spatial data efficiently. In essence, Trove’s hashmap solutions offer a versatile toolkit for Java developers seeking top-tier performance and memory optimization in their projects.
Trove’s unique features:
- Specialized for Primitives: Trove hashmaps handle primitive data types directly, boosting performance and memory efficiency;
- Memory Efficiency: Trove excels in memory optimization, crucial for applications where minimizing overhead matters;
- High-Performance Access: Trove ensures rapid data retrieval, ideal for high-throughput, low-latency scenarios;
- Versatile Applications: Trove’s hashmaps find use in finance, gaming, and geospatial systems, thanks to their specialized design;
- Reduced Cache Misses: Trove’s optimizations reduce cache misses, enhancing performance in data-intensive tasks;
- Open-Source and Community-Backed: Trove is open-source with an active community for ongoing support and development;
- Customization Options: Trove offers customization for tailored hashmap solutions;
- Reliable Data Management: Trove’s hashmaps deliver dependable data storage and retrieval for critical applications.
Here are some real-world examples of how these qualities make Trove an invaluable tool in various domains:
- Financial Systems: Trove’s hashmap solutions streamline numerical data management in the finance industry, aiding algorithmic trading platforms for swift and reliable financial data access;
- Gaming Engines: Trove’s hashmaps excel in gaming applications, ensuring fast data access and minimal memory usage, crucial for seamless gameplay;
- Geospatial Systems: Trove’s specialized hashmaps efficiently handle geospatial data, enhancing search, retrieval, and processing in GIS and mapping apps;
- Scientific Computing: Researchers benefit from Trove’s performance in large dataset handling and complex calculations for scientific computing and data analysis;
- In-Memory Caching: Trove hashmaps speed up in-memory caching systems, reducing costly database queries and boosting system performance;
- Big Data Processing: Trove’s hashmaps enhance big data processing by managing intermediate results, counters, and aggregations in data workflows;
- Embedded Systems: Trove’s memory-efficient design suits IoT devices, embedded controllers, and resource-constrained environments, optimizing memory usage.
Performance Benchmarks and Comparisons
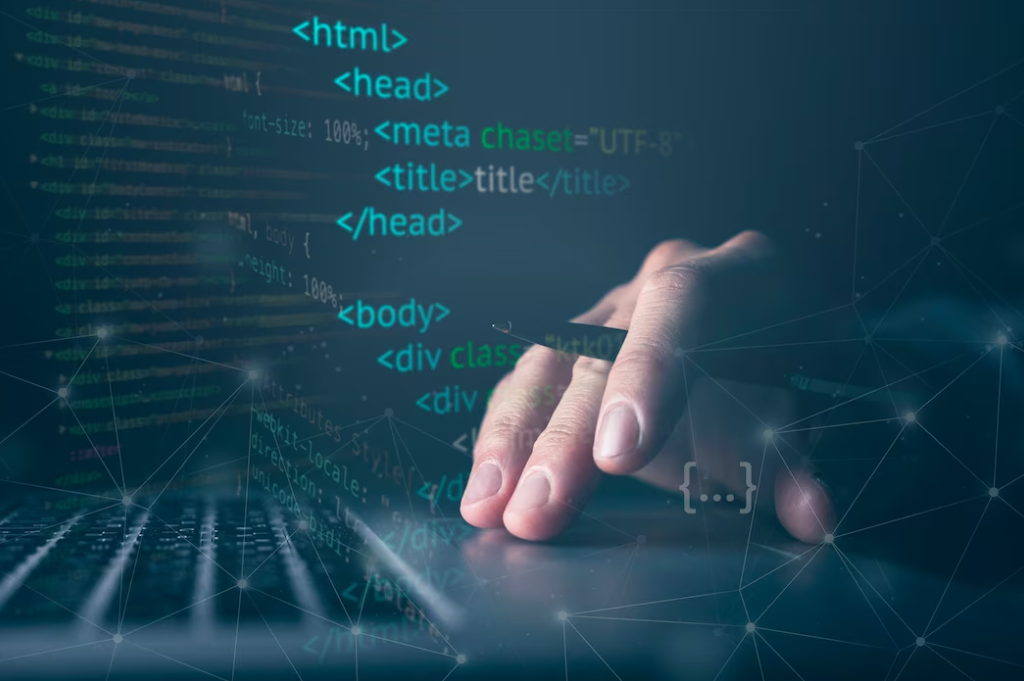
In the realm of data structures, hashmap implementations play a crucial role in optimizing the storage and retrieval of key-value pairs. When it comes to choosing the right hashmap for your specific use case, comprehensive performance benchmarks and comparisons are indispensable. In this article, we embark on an illuminating journey through the world of hashmap implementations, focusing on five prominent contenders:
- JDK’s Built-in Hashmap: We dive deep into Java Development Kit’s standard hashmap to establish a baseline for comparison;
- Fastutil: An in-depth analysis of Fastutil’s hashmap implementation, exploring its unique features and performance characteristics;
- Goldman Sachs HPPC: A close examination of Goldman Sachs High-Performance Primitive Collections hashmap, highlighting its suitability for demanding scenarios;
- Koloboke: We dissect Koloboke’s hashmap implementation, uncovering its strengths and weaknesses in various use cases;
- Trove: A thorough evaluation of Trove’s hashmap, shedding light on its advantages and potential trade-offs.
Conclusion
The exploration of Large Hashmap implementations—JDK, Fastutil, Goldman Sachs HPPC, Koloboke, and Trove—reveals a diverse array of options to enhance data management. Each library possesses unique strengths tailored to various use cases.
JDK Hashmap offers a reliable, general-purpose foundation, along with compatibility with standard Java libraries. Fastutil specializes in primitive data types, delivering exceptional memory efficiency and performance, particularly for numerical and data-intensive tasks. Goldman Sachs HPPC excels in high-throughput, low-latency scenarios, making it an ideal choice for rapid data processing. Koloboke stands out with its memory efficiency and speed, optimizing data storage and retrieval. Trove provides specialized features designed for specific use cases, especially in high-concurrency environments.
Selecting the right Large Hashmap depends on the project’s unique requirements, whether it’s memory efficiency, speed, or specialized functions.